fig.cap
is designed to take a single argument, and therefore we are going to have to programmatically generate new code chunks for each of the three plots you want. As explained here, we can use the knit_expand
function. I have amended the example provided to include the subfigure captions, and a way to print plots from a list.
I have written the function splitSubFig
which will limit the number of subfigures per plot. If we go over this number, it will create a new page. Note, you will need to use the results="asis"
in the chunk header creating the figures:
---
output:
pdf_document: default
header-includes:
- \usepackage{subfig}
---
```{r setup, include=FALSE}
knitr::opts_chunk$set(echo = F)
library(ggplot2)
library(knitr)
# Function to create a separate chunk to generate a plot for each item in list
splitSubFig <- function(plots, caption, maxlength) {
knitr::opts_knit$set(progress = FALSE, verbose = FALSE)
n <- length(plots)
numPages <- ceiling(n / maxlength)
splitPlots <- split(plots, rep(1:ceiling(n/maxlength), each=maxlength)[1:n])
for (page in 1:numPages) {
cat(
knit(text=knit_expand(
text=(
"```{r {{caption}}{{page}}, fig.cap='{{caption}}: {{page}}', fig.subcap=LETTERS[1:length(splitPlots[page])], out.width='8cm', fig.ncol=2, echo = FALSE, message = FALSE}
for(i in splitPlots[page]){
for(plots in i){
print(plots)
}
}
```"),
caption = caption,
page = LETTERS[page])))
}
}
```
```{r, results="asis"}
plots <- list(ggplot(data.frame(a = runif(50)), aes(x=a)) + geom_histogram(bins=10, fill = "red"),
ggplot(data.frame(a = runif(50)), aes(x=a)) + geom_histogram(bins=10),
ggplot(data.frame(a = runif(50)), aes(x=a)) + geom_histogram(bins=10),
ggplot(data.frame(a = runif(50)), aes(x=a)) + geom_histogram(bins=10),
ggplot(data.frame(a = runif(50)), aes(x=a)) + geom_histogram(bins=10),
ggplot(data.frame(a = runif(50)), aes(x=a)) + geom_histogram(bins=10, fill = "blue"),
ggplot(data.frame(a = runif(50)), aes(x=a)) + geom_histogram(bins=10),
ggplot(data.frame(a = runif(50)), aes(x=a)) + geom_histogram(bins=10),
ggplot(data.frame(a = runif(50)), aes(x=a)) + geom_histogram(bins=10),
ggplot(data.frame(a = runif(50)), aes(x=a)) + geom_histogram(bins=10))
splitSubFig(plots, caption = "Your Caption", maxlength = 6)
```
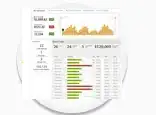
This function could certainly be improved, but it should be good for what you need!