I was able to find a way to parse the following string using JsonReader.SupportMultipleContent:
[{"a":"b"},{"c":"d"}],[{"e":"f"},{"g":"h"}]
Thanks @dbc for pointing me in the right direction (see Additional text encountered after finished reading JSON content).
Please note that this functionality is available only from Release 11.0.1 of Json.NET.
The following method uses a JsonTextReader
and returns a IEnumerable<KeyValuePair<string, string>>
:
private static IEnumerable<KeyValuePair<string, string>> ParseKeyValuePairs(string json)
{
using (var reader = new StringReader(json))
using (var jsonReader = new JsonTextReader(reader) { SupportMultipleContent = true })
{
JsonSerializer serializer = JsonSerializer.CreateDefault();
while (jsonReader.Read())
{
if (jsonReader.TokenType == JsonToken.Comment)
{
continue;
}
var dictionaries = serializer.Deserialize<List<Dictionary<string, string>>>(jsonReader);
foreach (KeyValuePair<string, string> keyValue in dictionaries.SelectMany(x => x))
{
yield return keyValue;
}
}
}
}
Using the code to generate a Dictionary<string, string>
:
string json = @"[{""a"":""b""},{""c"":""d""}],[{""e"":""f""},{""g"":""h""}]";
Dictionary<string, string> data = ParseKeyValuePairs(json).ToDictionary(x => x.Key, x => x.Value);
Result:
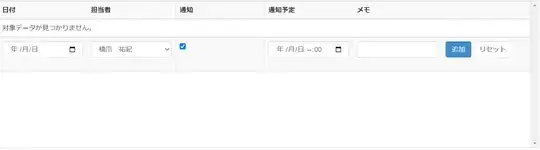