Try this way I have managed using
Dialogs
- A dialog is a small window that prompts the user to make a decision or enter additional information. A dialog does not fill the screen and is normally used for modal events that require users to take an action before they can proceed.
RecyclerView
- A flexible view for providing a limited window into a large data set.
SAMPLE CODE
MainActivity
public class MainActivity extends AppCompatActivity {
RelativeLayout myRelativeLayout;
TextView tvText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
myRelativeLayout = findViewById(R.id.myRelativeLayout);
tvText = findViewById(R.id.tvText);
myRelativeLayout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
showCustomDialog();
}
});
}
private void showCustomDialog() {
final Dialog myDialog = new Dialog(this);
myDialog.requestWindowFeature(Window.FEATURE_NO_TITLE);
RecyclerView myRecyclerView;
DataAdapter dataAdapter;
ArrayList<DataModel> arrayList = new ArrayList<>();
Button btnCancel;
// setting custom layout in out dialog
myDialog.setContentView(R.layout.custom_dialog_layout);
// findViewById for views inside dialog
myRecyclerView = myDialog.findViewById(R.id.MyRecyclerView);
btnCancel = myDialog.findViewById(R.id.btnCancel);
// programatically setting hight and witdh of dialog
Window window = myDialog.getWindow();
window.setLayout(WindowManager.LayoutParams.MATCH_PARENT, WindowManager.LayoutParams.WRAP_CONTENT);
// setting gravity of dialog so it will diaply in the center of screen
window.setGravity(Gravity.CENTER);
// setting TRANSPARENT Background to myDialog to display myDialog with rounded corner
myDialog.getWindow().setBackgroundDrawable(new ColorDrawable(Color.TRANSPARENT));
// adding data in to arrayList
arrayList.add(new DataModel("Very Small", false));
arrayList.add(new DataModel("Small", false));
arrayList.add(new DataModel("Normal", true));
arrayList.add(new DataModel("Large", true));
arrayList.add(new DataModel("Very Large", true));
// using DataAdapter.OnItemClickListener() getting result which item is selected in recyclerview
dataAdapter = new DataAdapter(this, arrayList, new DataAdapter.OnItemClickListener() {
@Override
public void onItemClick(DataModel item, int position) {
// setting result in a textview when user select recyclerview item
tvText.setText(item.getName());
}
});
// setting LayoutManager in myRecyclerView
myRecyclerView.setLayoutManager(new LinearLayoutManager(this));
// setting Adapter( in myRecyclerView
myRecyclerView.setAdapter(dataAdapter);
// click listner for btnCancel to dismiss myDialog
btnCancel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
myDialog.dismiss();
}
});
// used for display Dialog
myDialog.show();
}
}
layout.activity_main
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<RelativeLayout
android:id="@+id/myRelativeLayout"
android:layout_width="match_parent"
android:padding="5dp"
android:layout_height="wrap_content"
android:gravity="center">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentStart="true"
android:layout_centerInParent="true"
android:padding="5dp"
android:text="Language"
android:textColor="@android:color/black" />
<TextView
android:id="@+id/tvText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentEnd="true"
android:layout_centerInParent="true"
android:drawableEnd="@drawable/ic_arrow"
android:gravity="center"
tools:text="Deafult" />
</RelativeLayout>
</LinearLayout>
layout.custom_dialog_layout
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:cardCornerRadius="20dp"
app:cardElevation="5dp"
app:cardUseCompatPadding="true">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:layout_marginBottom="10dp"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:padding="10dp"
android:text="Text Size"
android:textColor="@android:color/black"
android:textSize="20sp" />
<View
android:layout_width="match_parent"
android:layout_height="2dp"
android:layout_marginTop="5dp"
android:background="@android:color/darker_gray" />
<android.support.v7.widget.RecyclerView
android:id="@+id/MyRecyclerView"
android:layout_width="match_parent"
android:layout_height="270dp" />
<Button
android:id="@+id/btnCancel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="10dp"
android:background="@drawable/round_button"
android:paddingStart="50dp"
android:paddingEnd="50dp"
android:text="Cancel"
android:textColor="#00b7ff" />
</LinearLayout>
</android.support.v7.widget.CardView>
DataAdapter
public class DataAdapter extends RecyclerView.Adapter<DataAdapter.ViewHolder> {
private Context context;
private ArrayList<DataModel> arrayList = new ArrayList<>();
private final OnItemClickListener listener;
public DataAdapter(Context context, ArrayList<DataModel> arrayList, OnItemClickListener listener) {
this.context = context;
this.arrayList = arrayList;
this.listener = listener;
}
private int lastCheckedPosition = -1;
@NonNull
@Override
public DataAdapter.ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(context).inflate(R.layout.custom_row_item, viewGroup, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull DataAdapter.ViewHolder viewHolder, int position) {
// here i'm changing the state of radio button based on user select
viewHolder.radioImage.setChecked(position == lastCheckedPosition);
viewHolder.tvTextName.setText(arrayList.get(position).getName());
// this is condition is used to hide line from thr last recyclerview item
if (position == arrayList.size()-1) {
viewHolder.bottomLine.setVisibility(View.GONE);
} else {
viewHolder.bottomLine.setVisibility(View.VISIBLE);
}
}
public interface OnItemClickListener {
void onItemClick(DataModel item, int position);
}
@Override
public int getItemCount() {
return arrayList.size();
}
public class ViewHolder extends RecyclerView.ViewHolder {
View bottomLine;
RadioButton radioImage;
TextView tvTextName;
public ViewHolder(@NonNull View itemView) {
super(itemView);
bottomLine = itemView.findViewById(R.id.bottomLine);
radioImage = itemView.findViewById(R.id.radioImage);
tvTextName = itemView.findViewById(R.id.tvTextName);
itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
arrayList.get(getAdapterPosition()).setSelected(true);
lastCheckedPosition = getAdapterPosition();
// getting result when user selecte radio button in your activity
listener.onItemClick(arrayList.get(lastCheckedPosition), lastCheckedPosition);
notifyDataSetChanged();
}
});
}
}
}
layout.custom_row_item
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="10dp"
android:layout_marginTop="10dp"
android:layout_marginEnd="10dp">
<TextView
android:id="@+id/tvTextName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentStart="true"
android:layout_centerInParent="true"
android:padding="5dp"
android:textColor="@android:color/black"
tools:text="TextName" />
<RadioButton
android:id="@+id/radioImage"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentEnd="true"
android:layout_centerInParent="true"
android:src="@drawable/ic__unchecked_radio_button" />
<View
android:id="@+id/bottomLine"
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_below="@id/tvTextName"
android:layout_marginTop="10dp"
android:background="@android:color/darker_gray" />
</RelativeLayout>
ic__unchecked_radio_button.xml
<vector xmlns:android="http://schemas.android.com/apk/res/android"
android:width="24dp"
android:height="24dp"
android:viewportWidth="24.0"
android:viewportHeight="24.0">
<path
android:fillColor="#FF000000"
android:pathData="M12,2C6.48,2 2,6.48 2,12s4.48,10 10,10 10,-4.48 10,-10S17.52,2 12,2zM12,20c-4.42,0 -8,-3.58 -8,-8s3.58,-8 8,-8 8,3.58 8,8 -3.58,8 -8,8z"/>
</vector>
ic_checked_radio_button.xml
<vector xmlns:android="http://schemas.android.com/apk/res/android"
android:width="24dp"
android:height="24dp"
android:viewportWidth="24.0"
android:viewportHeight="24.0">
<path
android:fillColor="#FF000000"
android:pathData="M12,7c-2.76,0 -5,2.24 -5,5s2.24,5 5,5 5,-2.24 5,-5 -2.24,-5 -5,-5zM12,2C6.48,2 2,6.48 2,12s4.48,10 10,10 10,-4.48 10,-10S17.52,2 12,2zM12,20c-4.42,0 -8,-3.58 -8,-8s3.58,-8 8,-8 8,3.58 8,8 -3.58,8 -8,8z"/>
</vector>
round_button.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:padding="10dp"
android:shape="rectangle">
<!-- this one is ths color of the Rounded Button -->
<solid android:color="#476e6e6e" />
<!-- this one is used to set radius of the Rounded Button -->
<corners android:radius="50dp" />
</shape>
OUTPUT
Please check this video(output of above code) https://www.youtube.com/watch?v=hGf-30Oh8OM
Screen-Shots
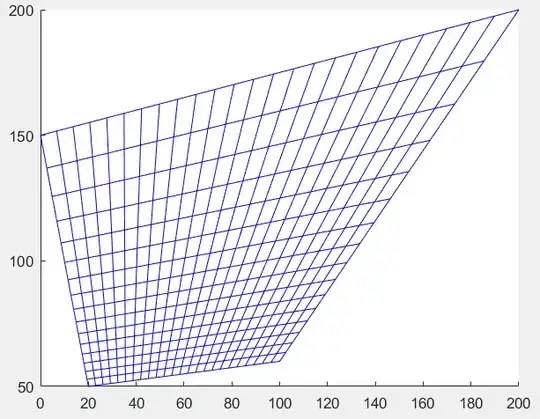
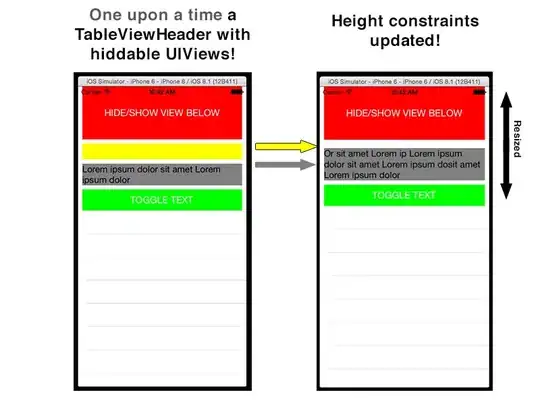
NOTE
You can also use PopupWindow
to achieve this
- This class represents a popup window that can be used to display an arbitrary view. The popup window is a floating container that appears on top of the current activity.
Here are some good article for it