This is actually a problem for which there is no good solution at the moment. The axis units are determined as they come from the input. So a solution is to predetermine the categorical order manually by plotting something in the correct order first and then remove it again.
import matplotlib.pyplot as plt
x = ['C9-U2', 'C10-U5', 'C10-U5', 'C11-U1', 'C11-U1']
y = ['J', 'C', 'H', 'J', 'H']
def unitsetter(xunits, yunits, ax=None, sort=True):
ax = ax or plt.gca()
if sort:
xunits = sorted(xunits)
yunits = sorted(yunits)
us = plt.plot(xunits, [yunits[0]]*len(xunits),
[xunits[0]]*len(yunits), yunits)
for u in us:
u.remove()
unitsetter(x,y)
plt.scatter(x,y)
plt.show()
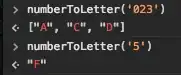
Here, sort
is set to True
, hence you get alphabetically sorted categories in both axes.
If you have a custom order you want the axis to obey, as is the case here (at least for the x axis) you would need to supply that order to the above function.
unitsetter(x, sorted(y), sort=False)
plt.scatter(x,y)
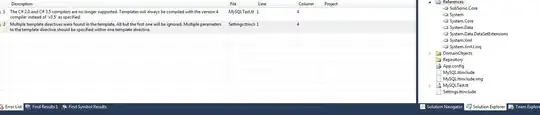