Depending on the size of your float number choose most suitable option:
var (
floatNumber float64 = 27.156633168032640
)
fmt.Println("as float32 with 'E' (decimal exponent) :", strconv.FormatFloat(floatNumber, 'E', -1, 32))
fmt.Println("as float64 with 'E' (decimal exponent) :", strconv.FormatFloat(floatNumber, 'E', -1, 64))
fmt.Println("as float32 with 'f' (no exponent) :", strconv.FormatFloat(floatNumber, 'f', -1, 32))
fmt.Println("as float64 with 'f' (no exponent) :", strconv.FormatFloat(floatNumber, 'f', -1, 64))
fmt.Println("with fmt.Sprint :", fmt.Sprint(floatNumber))
fmt.Println("with fmt.Sprintf :", fmt.Sprintf("%f", floatNumber))
The result is:
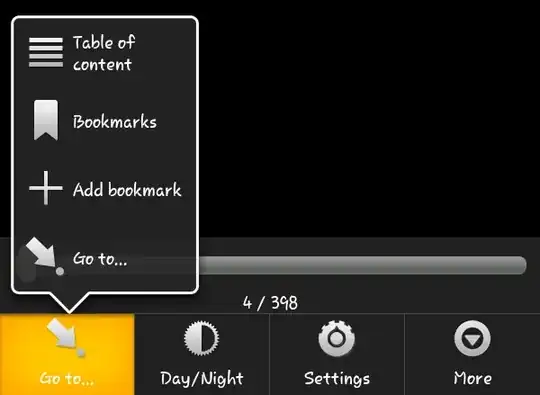
P.S. for better performance you should use strconv.FormatFloat()