I post an alternative approach which is not a true one liner but shows good performance as tested bellow.
The basic idea is same with @NathanOliver.
The idea is initializing a data set {"first", ..., "fourth"}
at a compile-time with static constexpr
qualifier to get a run-time performance gain:
In C++17 and over, std::string_view
is available and this has constexpr
ctors.
Thus it is natural to apply static constexpr std::array
of std::string_view
to improve the performance.
In addition, for such a small size data set ~10, naive linear search with cache locality of contiguous arrays sometimes beat other algorithms.
So I deliberately use std::find
here.
This is easily done using C++17's if statement with initializer as follows:
if(static constexpr
std::array<std::string_view, 4>
v = {"first", "second", "third", "fourth"};
std::find(v.cbegin(), v.cend(), s) != v.cend())
This can be summarized as the following compact and portable syntax sugar macro:
#include <tuple>
#include <array>
#include <string_view>
#include <algorithm>
#define IF_CONTAINS(str, ...) \
if(static constexpr \
std::array< \
std::string_view, \
std::tuple_size<decltype(std::make_tuple(__VA_ARGS__))>::value> \
v = {__VA_ARGS__}; \
std::find(v.cbegin(), v.cend(), str) != v.cend())
This macro IF_CONTAINS
works fine as if-statement as follows:
Live DEMO
std::string s = "first";
IF_CONTAINS(s, "first", "second", "third", "fourth") {
std::cout << s << " is found" << std::endl;
}
Performance Test 1
First, we test the performance of this approach with the above data set {"first", "second", "third", "fourth"}
.
Tests are done with Quick C++ Benchmark, gcc-8.2, C++17 and O3 optimization.
The results are as follows:


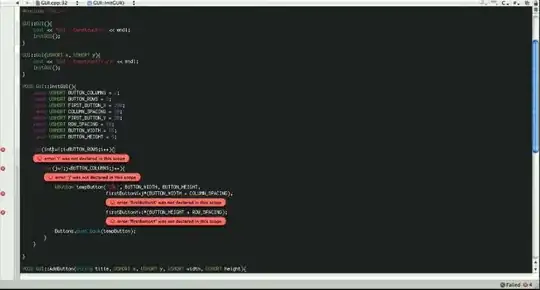
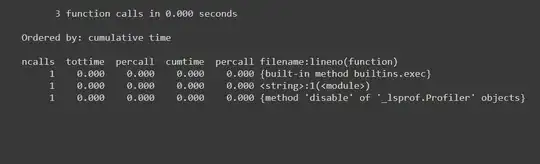
Performance Test 2
Finally, we test the performance with a data set {"1", ...,"10"}
.
The test code is same with @pawel_j 's one.
This test shows 2.7 times faster result than naive implementation:
Live DEMO
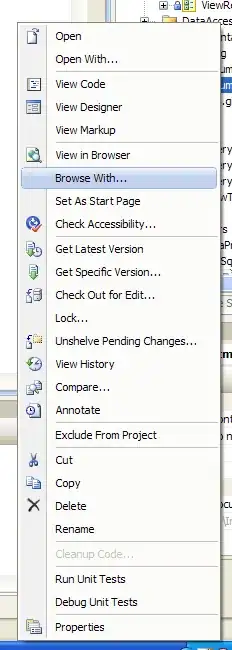