You just need to find the points where your x values (hereinafter called x
) are within your specified range, and each of your y values (y1
and y2
respectively) are equal. A naive approach might be
which(x <= xmax & x >= xmin & y1 == y2)
However, for the reasons detailed in Why are these numbers not equal?, that won't always work out. However, you can do something like this:
# Create an dataframe:
x <- seq(0, 10, 0.01)
y1 <- sin(x)
y2 <- cos(x)
df <- data.frame(x = x, y1 = y1, y2 = y2)
# Let's plot the curves
# I like to use the colorblind-friendly palette from
# Wong, Bang. 2011. "Points of view: Color blindness." Nature Methods 8:441.
wong_palette <- c("#e69f00", "#56b4e9")
# Plot the first curve
plot(x, y1, type = "l", xlab = "V1", ylab = "V2", col = wong_palette[1])
# Add the second
lines(x, y2, col = wong_palette[2])
# What are the intersections?
equivalent <- function(x, y, tol = 0.005) abs(x - y) < tol
xmin <- 3
xmax <- 8
intersection_indices <- which(equivalent(y1, y2) & x >= xmin & x <= xmax)
x[intersection_indices]
#> [1] 3.93 7.07
points(x[intersection_indices], y1[intersection_indices])
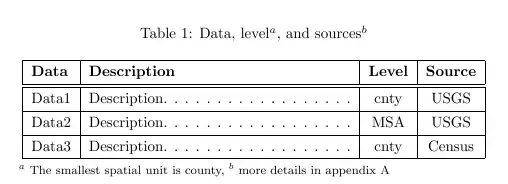
Created on 2018-11-18 by the reprex package (v0.2.1)
Note I did this with sample data I created myself, as your provided code snippet isn't quite sufficient to provide sample data. For future reference to write great questions, you may want to check out How to make a great R reproducible example.