Well, for others with the same issue, let me share my solution initially shared here: https://github.com/laravel/framework/issues/27729#issuecomment-1442976348
There are a lot of similar related issues what turns that very tricky.
I think here is the right thread to share what I found.
For instance, I'm working in a legacy system that was updated from Laravel 5.2 to 5.5 a few months ago, so keep in mind that your issue might be different.
Initially I thought that it could be due the settings of the middlewares, more about that in the links below:
After a few hours without success or anything really helpful, I decided to dig the old code with Laravel 5.2.
Well, they changed the behavior of ValidatesRequests
trait. Not only, but mainly the function validate
:
// 5.5
public function validate(Request $request, array $rules,
array $messages = [], array $customAttributes = [])
{
$this->getValidationFactory()
->make($request->all(), $rules, $messages, $customAttributes)
->validate();
return $this->extractInputFromRules($request, $rules);
}
// 5.2 - I didn't look carefully, but it seems that wasn't changed until 5.4
public function validate(Request $request, array $rules, array $messages = [], array $customAttributes = [])
{
$validator = $this->getValidationFactory()->make($request->all(), $rules, $messages, $customAttributes);
if ($validator->fails()) {
$this->throwValidationException($request, $validator);
}
}
After figured out that, I went back to the docs and didn't find anything related to that.
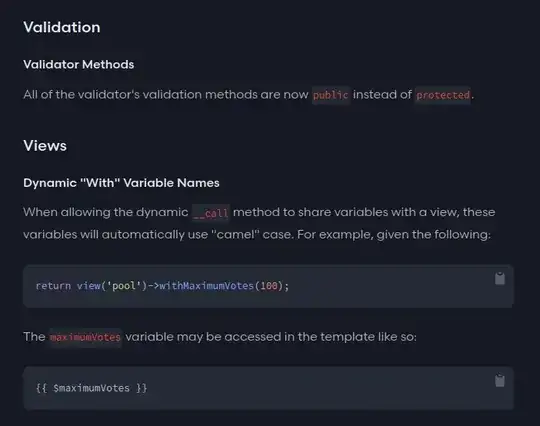
Anyway, it seems that after 5.5
if you want to send the errors and the inputs to the view, instead of only do return back();
, you will need to use something like return back()->withInput()->withErrors($exception->validator ?? '');
.
Alternatively,you can create a custom trait using the old code from 5.2
(perhaps combine both) and override the uses of ValidatesRequests
to CustomValidatesRequests
.
After recover the trait from 5.2
, the error messages came back to the system. :smiley:
Despite of that, keep in mind this might cause other issues, if possible it's better to use ->withInput()->withErrors($exception->validator ?? '');
.