You can use Angular's ng-deep if you want to affect the styles of its child components.
1.) On you ListingComponent, setup ng-deep and access the card container class
@Component({
selector: 'listing',
template: `<ng-content></ng-content>`,
styles: [
`
:host ::ng-deep .card-container { background-color: red; } // Keep in mind, to include :host which means this component as without it, this style will leak out affecting not only its child component class .card-container but also its siblings derived from another component
`
]
})
export class ListingComponent {}
2.) On you ListingDetailComponent, setup ng-deep and access the card container class
@Component({
selector: 'listing-detail',
template: `<ng-content></ng-content>`,
styles: [
`
:host ::ng-deep .card-container { background-color: green; }
`
]
})
export class ListingDetailComponent {}
3.) On you CardComponent, supposedly you have a card container class
@Component({
selector: 'card',
template: `<div class="card-container">Hi I'm Card</div>`,
})
export class CardComponent {}
4.) On your AppComponent, same with your structure
<listing>
<card></card>
</listing>
<listing-detail>
<card></card>
</listing-detail>
Here's the StackBlitz demo link for your reference
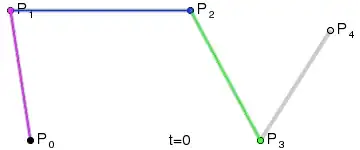
OR If you want to control the styling from the child component, you can do so by specifying :host-context and the parent's class.
Example:
1.) Specify the parent class that we will use to access from our child component (card)
<listing class="list-parent">
<card></card>
</listing>
<listing-detail class="list-detail-parent">
<card></card>
</listing-detail>
2.) On your child component (CardComponent), specify host-context on your styles. This way you can style your parent component in corresponds to their classes.
@Component({
selector: 'card',
template: `<div class="card-container">Hi I'm Card</div>`,
styles: [
`
:host-context(.list-parent) { background-color: red; }
:host-context(.list-detail-parent) { background-color: green; }
`
]
})
export class CardComponent {}