There's an annoying lack of quality Python packages for SDE integration. You have kind of an unusual setup, in that your random variable has an explicit dependence on your integrand (at least it appears that y
depends on p
). Because of this, it'll probably be hard to find a preexisting implementation that meets your needs.
Fortunately, the simplest method for SDE integration, Euler-Maruyama, is very easy to implement, as in the eulmar
function below:
from matplotlib import pyplot as plt
import numpy as np
def eulmar(func, randfunc, x0, tinit, tfinal, dt):
times = np.arange(tinit, tfinal + dt, dt)
x = np.zeros(times.size, dtype=float)
x[0] = x0
for i,t in enumerate(times[1:]):
x[i+1] = x[i] + func(x[i], t) + randfunc(x[i], t)
return times, x
You could then use eulmar
to integrate your SDE like so:
def func(x, t):
return 1 - 2*x
def randfunc(x, t):
return np.random.rand()
times,x = eulmar(func, randfunc, 0, 0, 500, 5)
plt.plot(times, x)
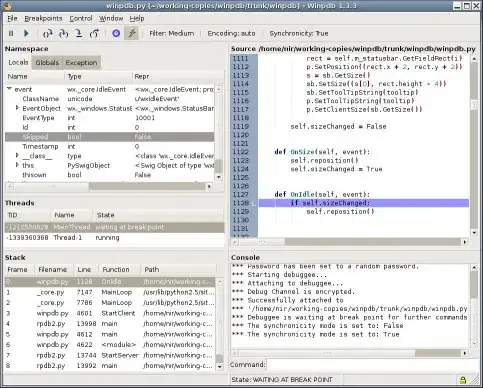
You'll have to supply your own randfunc
however. Like above, it should be a function that takes x
and t
as arguments and returns a single sample from your random variable y
. If you're having trouble coming up with a way to generate samples of y
, since you know the PDF
you can always use rejection sampling (though it does tend to be fairly inefficient).
Notes
- This is not a particularly efficient implementation of Euler-Maruyama. For example, the random samples are usually generated all at once (eg
np.random.rand(500)
). However, you can't pre-generate your random samples, since y
depends on p
.