my solution:
class ShadowImage
public class ShadowImage extends BitmapDrawable {
Bitmap bm;
static float shadowRadius = 4f;
static PointF shadowDirection = new PointF(2f, 2f);
int fillColor = 0;
@Override
public void draw(Canvas canvas) {
Rect rect = new Rect(0, 0, bm.getWidth(), bm.getHeight());
Log.i("TEST", rect.toString());
setBounds(rect);
Paint mShadow = new Paint();
mShadow.setAntiAlias(true);
mShadow.setShadowLayer(shadowRadius, shadowDirection.x, shadowDirection.y, Color.BLACK);
canvas.drawRect(rect, mShadow);
if(fillColor != 0) {
Paint mFill = new Paint();
mFill.setColor(fillColor);
canvas.drawRect(rect, mFill);
}
canvas.drawBitmap(bm, 0.0f, 0.0f, null);
}
public ShadowImage(Resources res, Bitmap bitmap) {
super(res, Bitmap.createScaledBitmap(bitmap, (int) (bitmap.getWidth()+shadowRadius*shadowDirection.x), (int) (bitmap.getHeight()+shadowRadius*shadowDirection.y), false));
this.bm = bitmap;
}
public ShadowImage(Resources res, Bitmap bitmap, int fillColor) {
super(res, Bitmap.createScaledBitmap(bitmap, (int) (bitmap.getWidth()+shadowRadius*shadowDirection.x), (int) (bitmap.getHeight()+shadowRadius*shadowDirection.y), false));
this.bm = bitmap;
this.fillColor = fillColor;
}
}
Activity:
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
LinearLayout root = (LinearLayout) findViewById(R.id.root_layout);
Bitmap bmp = BitmapFactory.decodeResource(getResources(), R.drawable.ic_launcher);
ShadowImage image = new ShadowImage(getResources(), bmp);
ShadowImage image2 = new ShadowImage(getResources(), bmp, Color.WHITE);
ImageView iv_normal = new ImageView(getApplicationContext());
iv_normal.setPadding(10, 10, 10, 10);
iv_normal.setImageBitmap(bmp);
ImageView iv_shadow = new ImageView(getApplicationContext());
iv_shadow.setPadding(10, 10, 10, 10);
iv_shadow.setImageDrawable(image);
ImageView iv_fill = new ImageView(getApplicationContext());
iv_fill.setPadding(10, 10, 10, 10);
iv_fill.setImageDrawable(image2);
LayoutParams params = new LayoutParams(LayoutParams.WRAP_CONTENT, LayoutParams.WRAP_CONTENT);
root.addView(iv_normal, params);
root.addView(iv_shadow, params);
root.addView(iv_fill, params);
root.setGravity(Gravity.CENTER);
}
Image: 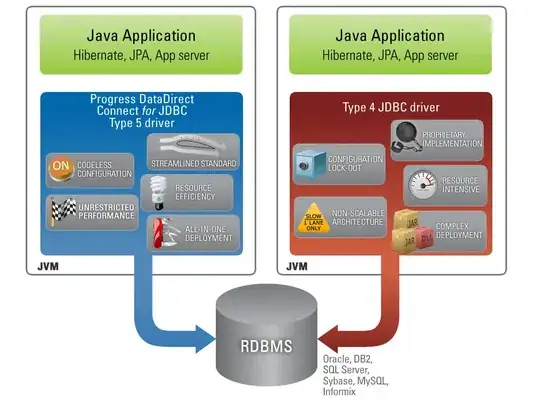