I've just created a package for add basic actions to the current keyboards .
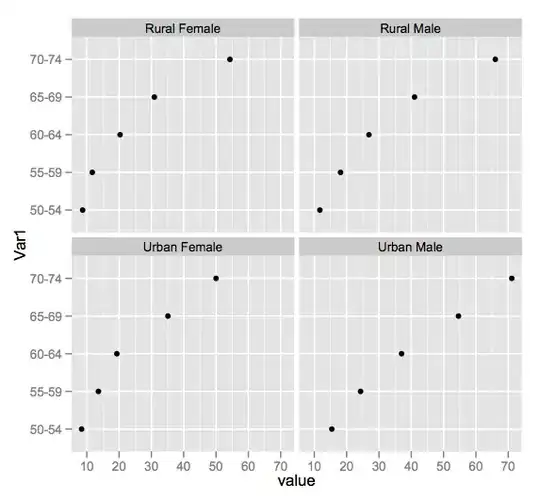
You can take a look here :
https://pub.dartlang.org/packages/keyboard_actions
Usage :
import 'package:flutter/material.dart';
import 'package:keyboard_actions/keyboard_actions.dart';
//...
FocusNode _nodeText1 = FocusNode();
FocusNode _nodeText2 = FocusNode();
FocusNode _nodeText3 = FocusNode();
FocusNode _nodeText4 = FocusNode();
FocusNode _nodeText5 = FocusNode();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Keyboard Actions Sample"),
),
body: FormKeyboardActions(
keyboardActionsPlatform: KeyboardActionsPlatform.ALL, //optional
keyboardBarColor: Colors.grey[200], //optional
nextFocus: true, //optional
actions: [
KeyboardAction(
focusNode: _nodeText1,
),
KeyboardAction(
focusNode: _nodeText2,
closeWidget: IconButton(
icon: Icon(Icons.close),
onPressed: () {},
),
),
KeyboardAction(
focusNode: _nodeText3,
onTapAction: () {
showDialog(
context: context,
builder: (context) {
return AlertDialog(
content: Text("Custom Action"),
actions: <Widget>[
FlatButton(
child: Text("OK"),
onPressed: () => Navigator.of(context).pop(),
)
],
);
});
},
),
KeyboardAction(
focusNode: _nodeText4,
displayCloseWidget: false,
),
KeyboardAction(
focusNode: _nodeText5,
closeWidget: Padding(
padding: EdgeInsets.all(5.0),
child: Text("CLOSE"),
),
),
],
child: Padding(
padding: const EdgeInsets.all(15.0),
child: SingleChildScrollView(
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
TextField(
keyboardType: TextInputType.number,
focusNode: _nodeText1,
decoration: InputDecoration(
hintText: "Input Number",
),
),
TextField(
keyboardType: TextInputType.text,
focusNode: _nodeText2,
decoration: InputDecoration(
hintText: "Input Text with Custom Close Widget",
),
),
TextField(
keyboardType: TextInputType.number,
focusNode: _nodeText3,
decoration: InputDecoration(
hintText: "Input Number with Custom Action",
),
),
TextField(
keyboardType: TextInputType.text,
focusNode: _nodeText4,
decoration: InputDecoration(
hintText: "Input Text without Close Widget",
),
),
TextField(
keyboardType: TextInputType.number,
focusNode: _nodeText5,
decoration: InputDecoration(
hintText: "Input Number with Custom Close Widget",
),
),
],
),
),
),
),
);
}