You need to create a new ExpandableObjectConverter
, supporting standard values.
Example
As an example, lets say, xlass A
has a property of type B
and we like to let the user to choose some predefined values for B
from a drop-down list in property grid.
After you select something from dropdown, the B
will be initialized and you still can edit C
:
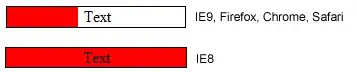
Here is the definition for A
and B
:
public class A
{
[TypeConverter(typeof(BConverter))]
public B B { get; set; }
}
public class B : ICloneable
{
[RefreshProperties(RefreshProperties.All)]
public string C { get; set; }
public object Clone()
{
return new B { C = this.C };
}
public override string ToString()
{
return C;
}
}
Here is the custom ExpandableObjectConverter
:
public class BConverter : ExpandableObjectConverter
{
B[] values = new B[] { new B { C = "Something" }, new B { C = "Something else" } };
public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType)
{
if (sourceType == typeof(string))
return true;
return base.CanConvertFrom(context, sourceType);
}
public override object ConvertFrom(ITypeDescriptorContext context,
System.Globalization.CultureInfo culture, object value)
{
var result = values.Where(x => $"{x}" == $"{value}").FirstOrDefault();
if (result != null)
return result.Clone();
return base.ConvertFrom(context, culture, value);
}
public override bool GetStandardValuesSupported(ITypeDescriptorContext c)
{
return true;
}
public override bool GetStandardValuesExclusive(ITypeDescriptorContext c)
{
return true;
}
public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext c)
{
return new StandardValuesCollection(values);
}
}