This is something to get you going (credit to this answer which I adapted).
We use annotate
to plot your labels
, on two different x-axis coords, this will function as our labels (so we need to shut off the actual labelling in the theme
).
First we create two vectors of the exact labels that we want in different colors.
dat <- data.frame(x = c(1,2,3,4,5,6), y = c(2,3,4,6,2,3))
breaks <- c(3,5)
labels_new1 <- c(NA, NA, 3, NA, 5, NA) # NA in order to skip that annotation
labels_new2 <- c(NA, NA, "(0.3)", NA, "(0.5)", NA)
Important parts:
coord_cartesian(xlim = c(0, 6), expand = FALSE) + # this will cut our plot properly
plot.margin = unit(c(1, 1, 1, 5), "lines") # this will give us some space on the left
Note that in coord_cartesian
defined like that we are actually cutting off the two annotations (notice that the two x values you see in the next part (-1, -0.5) are outside the xlim
range).
Plot object:
g1 <- ggplot() +
geom_point(data = dat, aes(x = x, y = y)) +
annotate(geom = "text", y = seq_len(nrow(dat)), x = -1, label = labels_new1, size = 4) +
#first the number add color = "blue" for example
annotate(geom = "text", y = seq_len(nrow(dat)), x = -0.5, label = labels_new2, size = 4, color = "red") +
#second the parenthesis (colored in red)
coord_cartesian(xlim = c(0, 6), expand = FALSE) +
scale_y_continuous(breaks = breaks) +
#now lets shut off the labels and give us some left space in the plot
theme(plot.margin = unit(c(1, 1, 1, 5), "lines"),
axis.title.y = element_blank(),
axis.text.y = element_blank())
Finally:
g2 <- ggplot_gtable(ggplot_build(g1)) # convert to grob
g2$layout$clip[g2$layout$name == "panel"] <- "off" # clipping of the axes
# this will show the two annotations that we left off before
grid::grid.draw(g2)
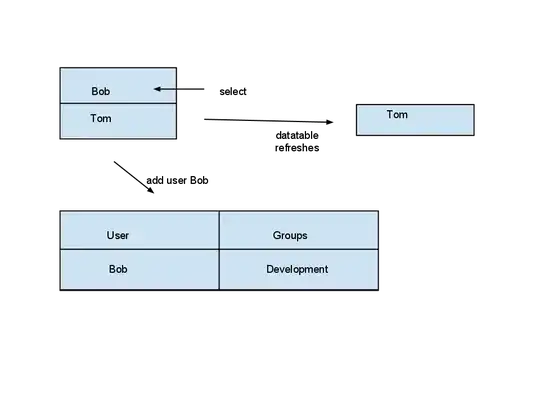
Remarks:
You can play around with x=-1
and x=-0.5
to move the two annotations, and with the last value in c(1, 1, 1, 5)
to give you more space on the left side.
labels_new1
and labels_new2
are very important, they are doing all the heavy work of what and where you want to show something.