The script below creates a small tkinter
app that draws something with turtle graphics when the Draw button is clicked. When the Save button was clicked, it saves the graphics into a bitmapped image file.
It uses the PIL (Python Image Library) module to convert the turtle graphics canvas into RGB image, and the converts that into a 1-bit per pixel bitmapped image.
Here's the turtlescreen.png
bitmapped image file it creates:
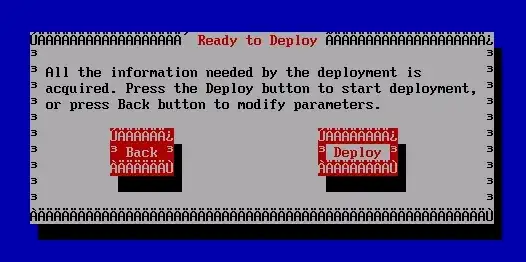
You've never indicated what the image format you want, so you may need to modify the script to create some other format—it currently creates a .png
format image. The extension of the image's filename determines this.
from PIL import ImageGrab
import tkinter as tk
import tkinter.messagebox as tkMessageBox
import turtle
WIDTH, HEIGHT = 500, 400
IMG_FILENAME = 'turtlescreen.png' # Extension determines file format.
def draw_stuff(canvas):
screen = turtle.TurtleScreen(canvas)
t = turtle.RawTurtle(screen.getcanvas())
t.speed(0) # Fastest.
t.pencolor('black')
t.hideturtle()
# Draw a snowflake.
size = 10
def branch(size):
for i in range(3):
for i in range(3):
t.forward(10.0*size/3)
t.backward(10.0*size/3)
t.right(45)
t.left(90)
t.backward(10.0*size/3)
t.left(45)
t.right(90)
t.forward(10.0*size)
# move the pen into starting position
t.penup()
t.forward(10*size)
t.left(45)
t.pendown()
# Draw 8 branches.
for i in range(8):
branch(size)
t.left(45)
print('done')
def getter(root, widget):
x = root.winfo_rootx() + widget.winfo_x()
y = root.winfo_rooty() + widget.winfo_y()
x1 = x + widget.winfo_width()
y1 = y + widget.winfo_height()
return ImageGrab.grab().crop((x, y, x1, y1))
def save_file(root, canvas, filename):
""" Convert the Canvas widget into a bitmapped image. """
# Get image of Canvas and convert it to bitmapped image.
img = getter(root, canvas).convert('L').convert('1')
img.save(IMG_FILENAME) # Save image file.
tkMessageBox.showinfo("Info", "Image saved as %r" % filename, parent=root)
# main
root = tk.Tk()
canvas = tk.Canvas(root, width=WIDTH, height=HEIGHT,
borderwidth=0, highlightthickness=0)
canvas.pack()
btn_frame = tk.Frame(root)
btn_frame.pack()
btn1 = tk.Button(btn_frame, text='Draw', command=lambda: draw_stuff(canvas))
btn1.pack(side=tk.LEFT)
btn2 = tk.Button(btn_frame, text='Save',
command=lambda: save_file(root, canvas, IMG_FILENAME))
btn2.pack(side=tk.LEFT)
btn3 = tk.Button(btn_frame, text='Quit', command=root.quit)
btn3.pack(side=tk.LEFT)
root.mainloop()