This situation happens when the parent and its child wants two different sizes; but the parent has no idea how it should align its child within its boundaries.
The following code doesn't give enough information to tell how to align the red box within the blue box:
Container(
color: Colors.blue,
width: 42,
height: 42,
child: Container(
color: Colors.red,
width: 24,
height: 24,
),
),
So while it could be centered as such:
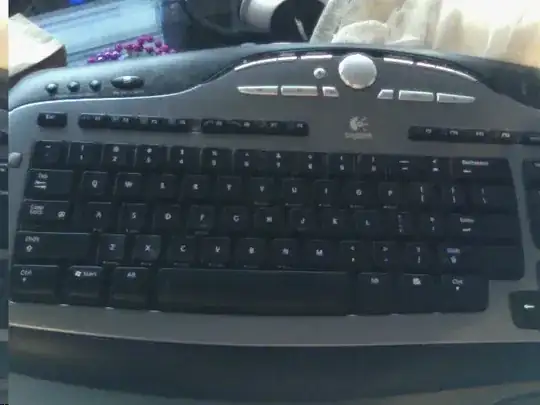
it would also be perfectly reasonable to expect the following alignment instead:
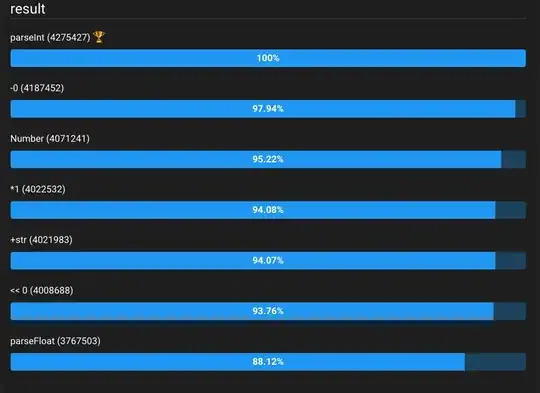
In that situation, since Flutter doesn't know how to align the red box, it will go with an easier solution:
Don't align the red box, and force it to take all the available space; which leads to:
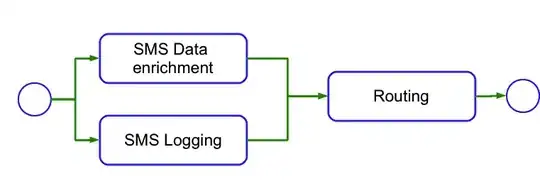
To solve this issue, the solution is to explicitly tell Flutter how to align the child within its parent boundaries.
The most common solution is by wrapping the child into an Align
widget:
Container(
color: Colors.blue,
width: 42,
height: 42,
child: Align(
alignment: Alignment.center,
child: Container(
color: Colors.red,
width: 24,
height: 24,
),
),
),
Some widgets such as Container
or Stack
will also offer an alignment
property to achieve similar behavior.