In terms of API, this is a very broad question. All graphics related APIs have transformations. So do the classes in the System.Numerics namespace, which are used mainly in SIMD operations. In geometry terms though, the answer is clear, you need to apply the correct transformation to all points. In this particular case it's reflection.
Some APIs support reflection directly, eg Vector2.Reflect :
var points=new[]{
new Vector2(0,0),
new Vector2(30,0),
new Vector2(30,-100),
new Vector2(0,-100),
};
var reflect_y=new Vector2(1,0);
var reflected = points.Select(p=>Vector2.Reflect(p,reflect_y))
.ToArray();
Printing out the reflected points produces :
0, 0
-30, 0
-30, -100
0, -100
In other cases one can calculate the transformation matrix and multiply each point by it with :
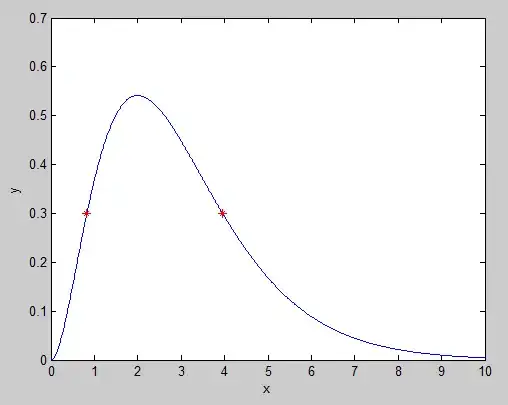
This article explains the math and shows the values to use for reflection along the X, Y axes or both. In this case the desired matrix is :
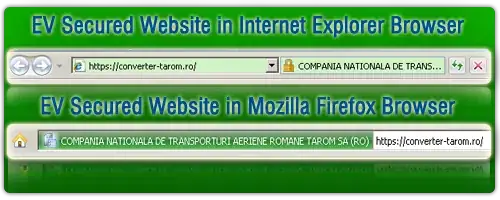
Transformations in computer graphics are applied as 3x2 matrices where the third column applies a transposition along each axis. In this case we don't want to move the result, so the third column contains 0s.
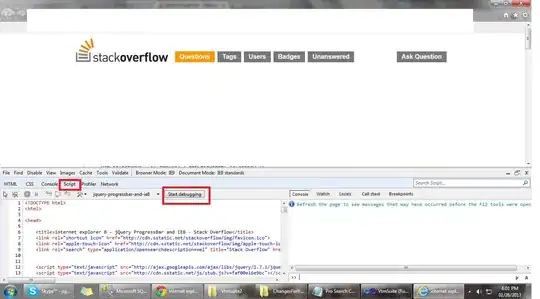
This time, Vector2.Transform is used instead of Reflect
:
var reflect_y=new Matrix3x2(-1,0,0,1,0,0);
var reflected = ( from point in points
select Vector2.Transform(point,reflect_y)
).ToArray();
In GDI+, a transformation is represented by a Matrix object. Reflect isn't available but can be replaced with Matrix.Scale when we want to reflect along the X or Y axes only. For example :
var m=new Matrix();
m.Scale(1,-1);
m.TransformVectors(points);
Will reflect an array of points by multiplying all X values by 1 and all Y values by -1.