I have never been able to get this to run as fast as i wanted so i came up with a different approach which complies to MVVM.
I created a virtualized stackpanel to contain textboxes that i create and add in code:
<ScrollViewer Grid.Column="2" Name="consoleScroll" Background="Black">
<ItemsControl ItemsSource="{Binding Log}">
<ItemsControl.ItemsPanel>
<ItemsPanelTemplate>
<VirtualizingStackPanel/>
</ItemsPanelTemplate>
</ItemsControl.ItemsPanel>
</ItemsControl>
</ScrollViewer>
Code:
public ObservableCollection<System.Windows.Controls.Control> Log
{
get { return (ObservableCollection<System.Windows.Controls.Control>)this.GetValue(LogProperty); }
set { this.SetValue(LogProperty, value); }
}
// Using a DependencyProperty as the backing store for Log. This enables animation, styling, binding, etc...
public static readonly DependencyProperty LogProperty =
DependencyProperty.Register(nameof(Log), typeof(ObservableCollection<System.Windows.Controls.Control>), typeof(ViewModel), new PropertyMetadata(default(ObservableCollection<System.Windows.Controls.Control>)));
//Code to call in a function
TextBox tb = new TextBox();
tb.Foreground = new SolidColorBrush(Colors.Hotpink);
tb.HorizontalContentAlignment = HorizontalAlignment.Left;
tb.Text = "my nice string";
Log.Add(tb);
The resulting console window works as expected and supports multi color. Needed to censor parts of it...
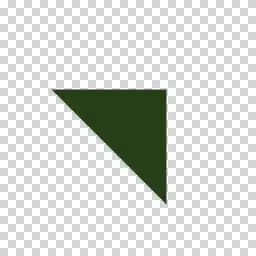