You seems to have a CSV
according RFC4180. There Definition of the CSV Format states:
Fields containing line breaks (CRLF), double quotes, and commas should be enclosed in double-quotes. For example:
"aaa","b CRLF
bb","ccc" CRLF
zzz,yyy,xxx
This is more complex than a simple CSV and cannot simply be read line by line then since not each linefeed means a new record. Try finding a CSV parser which supports RFC4180.
opencsv will be a such.
Example:
CSV.csv:
Field1,Field2,Field3
123,This is test column.,345
678,"This is test column.
This is next line",910
123,This is test column.,345
Code:
import java.io.FileOutputStream;
import java.io.FileReader;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import com.opencsv.CSVReader;
class ParseCSVToExcel {
public static void main(String[] args) throws Exception {
try (XSSFWorkbook workbook = new XSSFWorkbook();
FileOutputStream out = new FileOutputStream("Excel.xlsx");
FileReader in = new FileReader("CSV.csv")) {
CellStyle cellStyle = workbook.createCellStyle();
cellStyle.setWrapText(true);
Sheet sheet = workbook.createSheet("FromCSV");
Row row = null;
Cell cell = null;
int r = 0;
int maxC = 0;
CSVReader reader = new CSVReader(in);
String [] nextLine;
while ((nextLine = reader.readNext()) != null) {
row = sheet.createRow(r++);
int c = 0;
for (String field : nextLine) {
cell = row.createCell(c++);
cell.setCellValue(field);
cell.setCellStyle(cellStyle);
}
if (c > maxC) maxC = c;
}
for (int c = 0; c < maxC; c++) {
sheet.autoSizeColumn(c);
}
workbook.write(out);
}
}
}
Result:
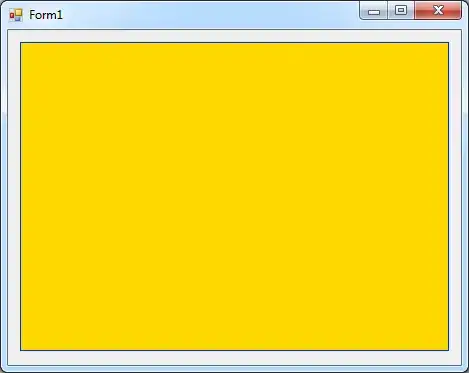
Using Apache Commons CSV would be another possibility.
Same CSV.csv
as above.
Code:
import java.io.FileOutputStream;
import java.io.FileReader;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.commons.csv.CSVRecord;
import org.apache.commons.csv.CSVFormat;
class ParseCSVToExcelApacheCommonsCSV {
public static void main(String[] args) throws Exception {
try (XSSFWorkbook workbook = new XSSFWorkbook();
FileOutputStream out = new FileOutputStream("Excel.xlsx");
FileReader in = new FileReader("CSV.csv")) {
CellStyle cellStyle = workbook.createCellStyle();
cellStyle.setWrapText(true);
Sheet sheet = workbook.createSheet("FromCSV");
Row row = null;
Cell cell = null;
int r = 0;
int maxC = 0;
for (CSVRecord record : CSVFormat.RFC4180.parse(in)) {
row = sheet.createRow(r++);
int c = 0;
for (String field : record) {
cell = row.createCell(c++);
cell.setCellValue(field);
cell.setCellStyle(cellStyle);
}
if (c > maxC) maxC = c;
}
for (int c = 0; c < maxC; c++) {
sheet.autoSizeColumn(c);
}
workbook.write(out);
}
}
}
Same result as above.