You can achieve it using Newtonsoft.Json.Linq.JObject
like following code.
var response = "{\"C0001\":{\"Balance\":3.01,\"CardCode\":\"C0001\",\"CardName\":\"Mubarik\",\"PriceLevel\":\"PL1\",\"Status\":true}}";
JObject search = JObject.Parse(response);
RootObject yourObject = search["C0001"].ToObject<RootObject>();
Online Demo
Output
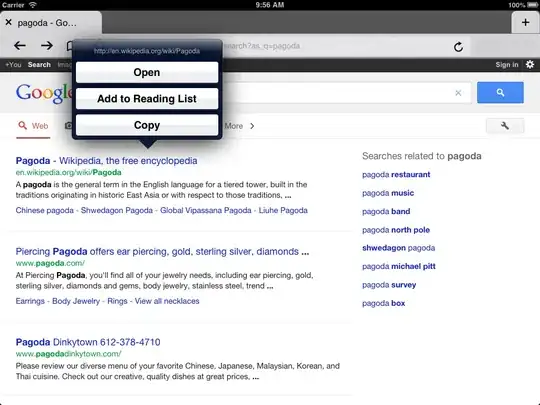
To read more about partial JSON fragment deserialization you can check here
EDIT:
what about this response? var bp =
"{\"C0001\":{\"Balance\":3.01,\"CardCode\":\"C0001\",\"CardName\":\"Mubarik\",\"PriceLevel\":\"PL1\",\"Status\":true},\"C0002\":{\"Balance\":1.03,\"CardCode\":\"C0001\",\"CardName\":\"Richie
Rich\",\"PriceLevel\":\"PL2\",\"Status\":true}}" – Mubah Mohamed
As per the format in the comment where you're getting multiple object in JSON with different ID, you can try like following to convert it into a list of RootObject
.
var response = "{\"C0001\":{\"Balance\":3.01,\"CardCode\":\"C0001\",\"CardName\":\"Mubarik\",\"PriceLevel\":\"PL1\",\"Status\":true},\"C0002\":{\"Balance\":1.03,\"CardCode\":\"C0001\",\"CardName\":\"Richie Rich\",\"PriceLevel\":\"PL2\",\"Status\":true}}";
JObject search = JObject.Parse(response);
IList<JToken> results = search.Children().ToList();
List<RootObject> searchResults = new List<RootObject>();
foreach (JToken result in results)
{
RootObject searchResult = result.First.ToObject<RootObject>();
searchResults.Add(searchResult);
}
Online Demo