The way you are loading your XIB creates two UIView
objects, where you may be thinking it creates only one.
You can easily see this using Debug View Hierarchy
Here, the Blue view is the view added to Storyboard, with constraints set normally (in this case, 40-pts from each side, height of 100, and centered vertically).
The Red view is the view you work with when designing your XIB, and it has a label with black background, constrained to 0
on all four sides.
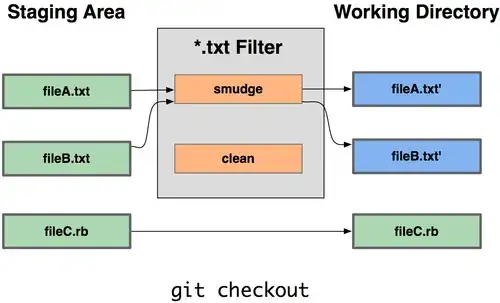
Your XIB class is UIView
-- so it is its own view... When you added a UIView
to your storyboard and set its class to CustomLabel
, that becomes the "root" view. In your code, you are adding view
as a subview... which should give you the clue that you now have two UIView
objects.
What's happened now is that the CustomLabel
itself (which is a UIView
) uses the constraints you set in storyboard, but the view
subview does not have constraints set.
If you change your loading code to this (I changed your IBOutlet
names, because adding "view" as a subview to another "view" can become very confusing):
class CustomLabel: UIView {
@IBOutlet var theView: UIView!
@IBOutlet weak var thelabel: UILabel!
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
print("CustomLabel")
Bundle.main.loadNibNamed("CustomLabel", owner: self, options: nil)
self.addSubview(self.theView)
self.theView.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activate([
self.theView.topAnchor.constraint(equalTo: self.topAnchor, constant: 0.0),
self.theView.bottomAnchor.constraint(equalTo: self.bottomAnchor, constant: 0.0),
self.theView.leadingAnchor.constraint(equalTo: self.leadingAnchor, constant: 0.0),
self.theView.trailingAnchor.constraint(equalTo: self.trailingAnchor, constant: 0.0),
])
}
}
Now, your code loads the view from the XIB, adds it as a subview of CustomLabel
(which, again, is a UIView
itself), and sets up the constraints as you'd expect, giving this result:
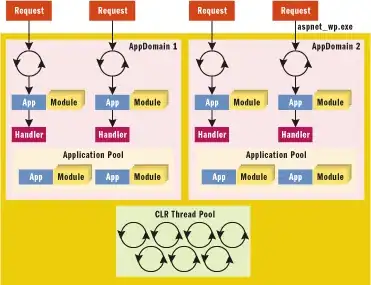
As you can see, theView
which has been added as a subview (red in this image), is now constrained to its superview - the blue CustomLabel
.