So as I said, I'm doing a project. My project is a system to manage parent meeting's day in school.
Those are my tables, relationships, and such :
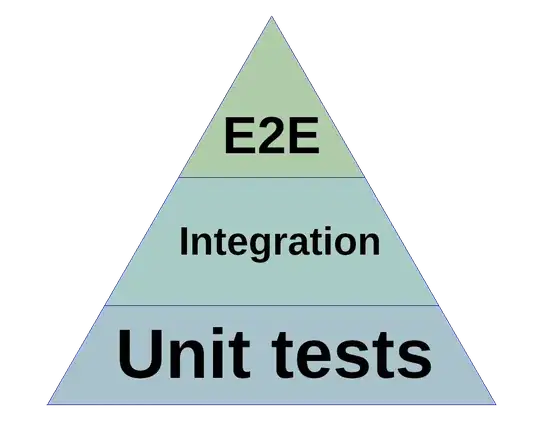
My goal was to create a "getAllDates" function in my API.
In my DB, the Dates table is where all the "teacher-parent meetings" information stored. As a result of that, my Dates table contains A bunch of foreign keys, that
in my API code are translated to instances of other models in the Dates model, as seen here:
namespace ProjAPI.Models
{
public class Date
{
public DateTime DateInfo;
public Student Student;
public Teacher Teacher;
public Classroom Classroom;
public int Status, Id;
}
}
As you imagine, each of the instances have properties of its own, properties i need to supply him. That's when my problem begun.
So let's take a look on the other models:
User Model
namespace ProjAPI.Models
{
public class User
{
public string Name, LastName, Address, Phone, Pass, Email, Id;
public int Role, Status;
}
}
Student Model
namespace ProjAPI.Models
{
public class Student : User
{
public string YearAndClass;
}
}
Teacher Model
namespace ProjAPI.Models
{
public class Teacher : User
{
public Classroom Classroom;
}
}
Classroom Model
namespace ProjAPI.Models
{
public class Classroom
{
public Building Building;
public string Id;
public string Name;
}
}
Building Model
namespace ProjAPI.Models
{
public class Building
{
public string Id;
public int RoomNum;
}
}
So, I needed a query that would :
1. take all the information for the teacher and the student and will rename the columns
so i can access them in the code.
2. take all of the information about the classes, and distinct them so i can access each class separately.
3. take all of the information about the date.
In order to solve that, i created temporary tables within my query:
(
SELECT
Classes.ClassID as cID, Classes.ClassName as cName,
Buildings.BuildingID as bID, Buildings.RoomNum
FROM
Classes
FULL OUTER JOIN
Buildings
on
Classes.BuildingID = Buildings.BuildingID
) as cb,
cb is a temp table that represent the class in my Dates table.
(
SELECT
Buildings.RoomNum as tcRoomNum,
Classes.ClassID as tcID, Classes.BuildingID as tcbID,
Classes.ClassName,ut.*
FROM
Classes,Buildings,
(
SELECT
UserID as tID, Name as tName, LastName as tLastName,
Status as tStatus, Role as tRole, Phone as tPhone,
Email as tEmail, Address as tAddress,Password as tPassword,
ClassID as clID
FROM
Users
LEFT JOIN
Teachers
ON
UserID = TeacherID
) as ut
WHERE
ut.clID=Classes.ClassID
AND Classes.BuildingID = Buildings.BuildingID
) as utcb,
utcb is a temp table to represent the teacher in my Dates table.
(
SELECT
UserID as sID, Name as sName, LastName as sLastName,
Status as sStatus, Role as sRole, Phone as sPhone,
Email as sEmail, Address as sAddress, Password as sPassword,
YearAndClass
FROM
Users
LEFT JOIN
Students
ON
UserID = StudentID
) as us
us is a temp table to represent the student in my Dates table.
Now, when I had created the temp tables, i accessed them and filter correctly:
SELECT
DateInfo,DateID,Dates.Status as dStatus,us.*,utcb.*,cb.*
FROM
Dates,cb,utcb,us
WHERE
Dates.StudentID = us.sID
AND Dates.TeacherID = utcb.tID
AND Dates.ClassID = cb.cID
I know I did it bad and inefficient, but I did it alone and it was tough and I'm proud of my self.
If anyone have any suggestions to upgrade this query feel free to let me know.