If a single answer is allowed to be checked among the list of answers, you need to use RadioButtons
If multiple answers are allowed to be checked, you need to use CheckBoxes or ToggleButtons
Here is a snippet of using ToggleButtons which simulates the button as clicked
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<ToggleButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/select"
android:textOff="Unselected"
android:textOn="Selected" />
<ToggleButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/select"
android:textOff="Unselected"
android:textOn="Selected" />
<ToggleButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/select"
android:textOff="Unselected"
android:textOn="Selected" />
<ToggleButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/select"
android:textOff="Unselected"
android:textOn="Selected" />
</LinearLayout>
and create a drawable for the background selection
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@color/checked_button" android:state_checked="true" />
<item android:drawable="@color/unchecked_button" android:state_checked="false" />
</selector>
colors
<resources>
<?xml version="1.0" encoding="utf-8"?>
...
<color name="checked_button">#989898</color>
<color name="unchecked_button">#f8f8f8</color>
</resources>
Java:
ToggleButton button1 = findViewById(R.id.btn1);
ToggleButton button2 = findViewById(R.id.btn2);
ToggleButton button3 = findViewById(R.id.btn3);
ToggleButton button4 = findViewById(R.id.btn4);
button1.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if (isChecked) {
// Button1 is checked
} else {
// Button1 is unchecked
}
}
});
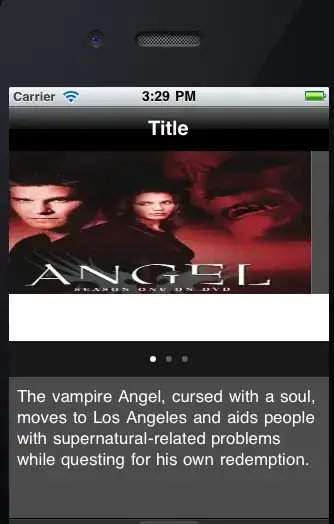