Ok, the problem was not in the plt.scatter
but in the plt.colorbar
. This was not clear from the initial question because you didn't include the second command before. It took some comments to find the problem.
The problem was that you were not creating any color map but was trying to show it without having any mappable numerical values to your color map. The solution below does the following things:
- First find the unique colors in your color array using
np.unique
.
- Then, create a custom color map using
ListedColormap
from your defined colors. This was inspired by the solution provided here. I upvoted it, so should you.
- Mapping the strings (colors) to integers. I found how to do this from here. I upvoted it, so should you.
- Last but not the least, use the mapped values as colors with the custom created color map.
Here is a complete solution:
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.colors import ListedColormap
classes = 2
zones = (['FEF', 'IT'])
X = np.array([[-1.61160406, 0.06705226],
[-2.34304523, 0.19353161],
[-4.39162911, -0.15401544],
[-1.9107751 , 0.67541723],
[-1.76792646, 0.71884401]])
y = np.array(['c', 'mediumpurple', 'mediumpurple', 'c', 'c'], dtype='<U12')
cmap = ListedColormap(np.unique(y))
# print (np.unique(y))
# ['c' 'mediumpurple']
# Mapping the colors to numbers
dics = {k: v for v, k in enumerate(sorted(set(y)))}
y_mapped = [dics[x] for x in y]
# print (y_mapped)
# [0, 1, 1, 0, 0]
plt.scatter(X[:, 0], X[:, 1], c=y_mapped, cmap=cmap)
plt.colorbar(ticks=range(classes)).set_ticklabels(zones)
plt.show()
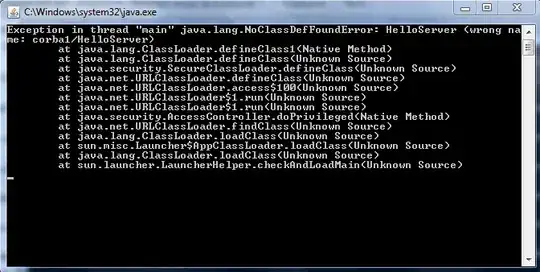