These instruction are for a CSV file but should work for an excel file as well.
If the repository is private, you might need to create a personal access token as described in "Creating a personal access token" (pay attention to the permissions especially if the repository belongs to an organisation).
- Click the "raw" button in GitHub. Here below is an example from https://github.com/udacity/machine-learning/blob/master/projects/boston_housing/housing.csv:
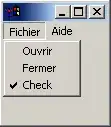
If the repo is private and there is no ?token=XXXX
at the end of the url (see below), you might need to create a personal access token and add it at the end of the url. I can see from your URL that you need to configure your access token to work with SAML SSO, please read About identity and access management with SAML single sign-on and Authorizing a personal access token for use with SAML single sign-on
- Copy the link to the file from the browser navigation bar, e.g.:
https://raw.githubusercontent.com/udacity/machine-learning/master/projects/boston_housing/housing.csv
- Then use code:
import pandas as pd
url = (
"https://raw.githubusercontent.com/udacity/machine-learning/master"
"/projects/boston_housing/housing.csv"
)
df = pd.read_csv(url)
In case your repo is private, the link copied would have a token at the end:
https://raw.githubusercontent.com/. . ./my_file.csv?token=XXXXXXXXXXXXXXXXXX