Yes.
Convert image into HSV color space.
Calculate yellow range in HSV (from Scalar to Scalar).
Create binary mask for yellow: inRange.
Call add with mask from (3) for your HSV image and cv::Scalar(5, 0, 0)
Convert Result to RGB.
Example:
cv::Mat rgbImg = cv::imread("src.jpg", cv::IMREAD_COLOR);
cv::Mat hsvImg;
cv::cvtColor(rgbImg, hsvImg, cv::COLOR_BGR2HSV);
cv::Mat threshImg;
cv::inRange(hsvImg, cv::Scalar(20, 100, 100), cv::Scalar(30, 255, 255), threshImg);
cv::imwrite("thresh.png", threshImg);
cv::add(hsvImg, cv::Scalar(5, 0, 0), hsvImg, threshImg);
cv::cvtColor(hsvImg, rgbImg, cv::COLOR_HSV2BGR);
cv::imwrite("res.png", rgbImg);
And pictures:
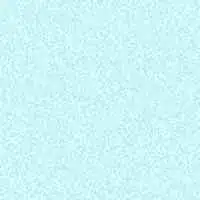