Solution for negative duration calculations
All these answers are pretty good but they didn't help me when I had a user select a range of times in my app. In order to calculate the total duration of the specified time period, I tried all the solutions which work pretty well but fail in certain scenarios. The scenarios are:
- When the start time comes after the end time (i.e- when the duration is supposed to be over 12 hours)
- When the start time is before 12am at the night and end time is after that
And the code to overcome it is:
String durationFromTimeOfDay(TimeOfDay? start, TimeOfDay? end) {
if (start == null || end == null) return '';
// DateTime(year, month, day, hour, minute)
final startDT = DateTime(9, 9, 9, start.hour, start.minute);
final endDT = DateTime(9, 9, 10, end.hour, end.minute);
final range = DateTimeRange(start: startDT, end: endDT);
final hours = range.duration.inHours % 24;
final minutes = range.duration.inMinutes % 60;
final _onlyHours = minutes == 0;
final _onlyMinutes = hours == 0;
final hourText = _onlyMinutes
? ''
: '$hours${_onlyHours ? hours > 1 ? ' hours' : ' hour' : 'h'}';
final minutesText = _onlyHours
? ''
: '$minutes${_onlyMinutes ? minutes > 1 ? ' mins' : ' min' : 'm'}';
return hourText + minutesText;
}
It is important to note that you need to prefill the DateTime
for end TimeOfDay
with a day value which is greater than the same in start DateTime
. The other parameters (for year and month) can be anything you want.
This outputs a really nicely formatted string that is short, concise, and extremely legible
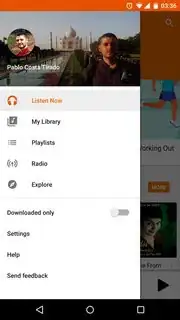
This, however, doesn't satisfy the requirement that the solution is devoid of conversion to DateTime. But at least it uses a different approach over the difference
method. And this makes the correct duration calculation more reliable in a few lines of code comparatively.