This answer about VB6 is pretty good, the implementation of the memento pattern and the way to refer to the properties through a type in VBA is what achieves the copying of the properties.
An object of type Employee with properties Salary
, Age
and RelevantExperience
is created. Then a new object, copying the old one with the function .Copy
is created. The new object initially has the same properties, but we may choose to change some of them. In the code below the Experience and the Age is changed, the Salary is not mentioned, thus it stays the same:
Dim newEmp As Employee
Dim oldEmp As Employee
Set newEmp = New Employee
With newEmp
.Salary = 100
.Age = 22
.RelevantExperience = 1
End With
Set oldEmp = newEmp.Copy
With oldEmp
'Salary is the same as in the NewEmp
.Age = 99
.RelevantExperience = 10
End With
This is the result:
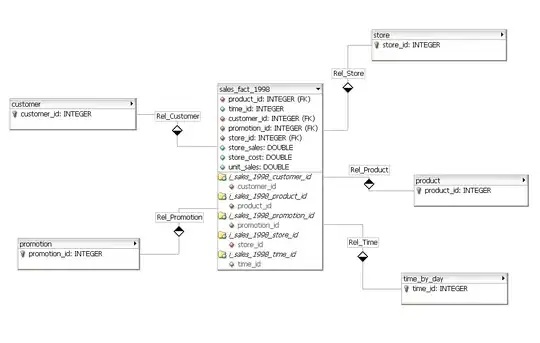
The old Employee has the same salary, "inherited" by the new employee, when he is copied. The Experience and the Age are different.
Full implementation
In a module:
Type MyMemento
Salary As Double
Age As Long
RelevantExperience As Long
End Type
Sub Main()
Dim newEmp As Employee
Dim oldEmp As Employee
Set newEmp = New Employee
With newEmp
.Salary = 100
.Age = 22
.RelevantExperience = 1
End With
Set oldEmp = newEmp.Copy
With oldEmp
'Salary is inherited, thus the same
.Age = 99
.RelevantExperience = 10
End With
Debug.Print "Salary"; vbCrLf; newEmp.Salary, oldEmp.Salary
Debug.Print "Experience"; vbCrLf; newEmp.RelevantExperience, oldEmp.RelevantExperience
Debug.Print "Age"; vbTab; vbCrLf; newEmp.Age, oldEmp.Age
End Sub
In a class module, called Employee
:
Private Memento As MyMemento
Friend Sub SetMemento(NewMemento As MyMemento)
Memento = NewMemento
End Sub
Public Function Copy() As Employee
Dim Result As Employee
Set Result = New Employee
Result.SetMemento Memento
Set Copy = Result
End Function
Public Property Get Salary() As Double
Salary = Memento.Salary
End Property
Public Property Let Salary(value As Double)
Memento.Salary = value
End Property
Public Property Get Age() As Long
Age = Memento.Age
End Property
Public Property Let Age(value As Long)
Memento.Age = value
End Property
Public Property Get RelevantExperience() As Long
RelevantExperience = Memento.RelevantExperience
End Property
Public Property Let RelevantExperience(value As Long)
Memento.RelevantExperience = value
End Property