Here's a shot at one:
shp <- structure(list(x = c(173L, 173L, 185L, 190L, 231L, 267L, 185L,
190L, 233L, 260L, 190L, 231L, 260L, 230L, 230L, 172L, 233L, 230L,
231L, 267L, 185L, 172L, 233L, 231L, 231L), y = c(299L, 299L,
321L, 360L, 361L, 377L, 321L, 360L, 363L, 309L, 360L, 361L, 309L,
322L, 322L, 378L, 363L, 322L, 391L, 377L, 321L, 378L, 363L, 391L,
361L), polygonID = c(2L, 4L, 5L, 3L, 6L, 7L, 2L, 5L, 7L, 6L,
2L, 3L, 4L, 5L, 6L, 2L, 3L, 4L, 3L, 6L, 4L, 3L, 6L, 7L, 5L)), class = "data.frame",
row.names = c(NA, -25L))
aa <- shp[ shp$polygonID == 2, ]
aa <- aa[ chull(aa[,1:2]), ]
aa
# x y polygonID
# 7 185 321 2
# 1 173 299 2
# 16 172 378 2
# 11 190 360 2
Now aa
is a 4-point polygon, ordered clockwise. Let's hard-code some calculations for now:
indm1 <- c(ind[-1], ind[1])
indp1 <- c(ind[length(ind)], ind[-length(ind)])
angles <- ((atan2(aa$y[indm1] - aa$y[ind], aa$x[indm1] - aa$x[ind]) -
atan2(aa$y[indp1] - aa$y[ind], aa$x[indp1] - aa$x[ind])) * 180 / pi) %% 360
cbind(indm1,ind,indp1)
# indm1 ind indp1
# [1,] 2 1 4
# [2,] 3 2 1
# [3,] 4 3 2
# [4,] 1 4 3
angles
# [1] 158.69530 29.33568 44.27478 127.69424
And let's see (I was initially perplexed that it was not visually-correlating until I realized that the aspect-ratio was off, ergo asp=1
).
plot(y~x, data=aa, type='l', asp=1)
with(aa, text(x-5, y, seq_len(nrow(aa)), col="red"))
with(aa, text(x+5, y, round(angles, 0)))
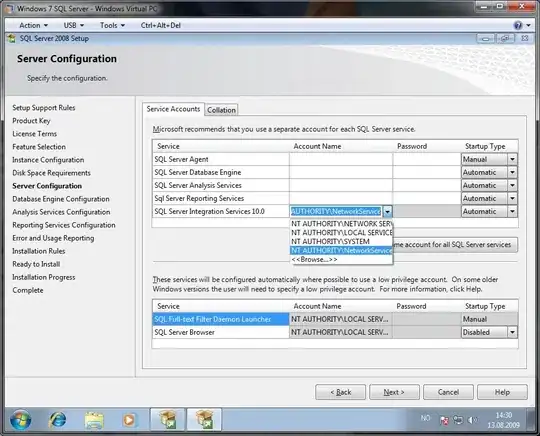
Okay, let's try to formalize this a little:
getangles <- function(aa) {
aa <- aa[chull(aa[,1:2]),]
ind <- seq_len(nrow(aa))
indm1 <- c(ind[-1], ind[1])
indp1 <- c(ind[length(ind)], ind[-length(ind)])
((atan2(aa$y[indm1] - aa$y[ind], aa$x[indm1] - aa$x[ind]) -
atan2(aa$y[indp1] - aa$y[ind], aa$x[indp1] - aa$x[ind])) * 180 / pi) %% 360
}
by(shp, shp$polygonID, getangles)
# shp$polygonID: 2
# [1] 158.69530 29.33568 44.27478 127.69424
# ------------------------------------------------------------
# shp$polygonID: 3
# [1] 130.91438 136.39718 133.60282 57.42594 81.65967
# ------------------------------------------------------------
# shp$polygonID: 4
# [1] 29.98564 54.83259 119.88349 155.29828
# ------------------------------------------------------------
# shp$polygonID: 5
# [1] 92.74183 81.42121 98.70294 87.13402
# ------------------------------------------------------------
# shp$polygonID: 6
# [1] 72.44870 111.95989 136.46880 157.38014 61.74247
# ------------------------------------------------------------
# shp$polygonID: 7
# [1] 71.70548 64.66388 43.63064
(There might be some issues with rounding/modulus, I'll leave it to you to beautify and verify the others.)