Your image shows that you want to edit line caps and line joins in all paths to be round.
Unfortunately you did not share representative example files, so I had to construct one myself with a mix of different cap and join styles and a path form reminding of yours:
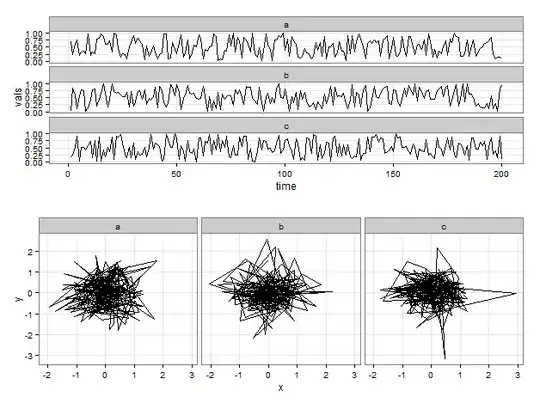
I'd propose for your task to make use of the generic PdfContentStreamEditor
from this answer as it does all the heavy lifting and we can concentrate on the task at hand.
Thus, what does our stream editor implementation have to do? It has to set cap and join styles to "round" and prevent these settings from being overridden. Looking into the PDF specification we see that cap and join styles are parameters of the current graphics state and can either be set directly using the J and j instructions respectively or via the LC and LJ entries in a Graphics State Parameter Dictionary.
Thus, we can implement our stream editor simply by first initializing cap and join style to round and then drop all J and j instructions and re-initialize cap and join styles after each graphics state gs instruction.
class PathMakeCapAndJoinRound : PdfContentStreamEditor
{
protected override void Write(PdfContentStreamProcessor processor, PdfLiteral operatorLit, List<PdfObject> operands)
{
if (start)
{
initializeCapAndJoin(processor);
start = false;
}
if (CAP_AND_JOIN_OPERATORS.Contains(operatorLit.ToString()))
{
return;
}
base.Write(processor, operatorLit, operands);
if (GSTATE_OPERATOR == operatorLit.ToString())
{
initializeCapAndJoin(processor);
}
}
void initializeCapAndJoin(PdfContentStreamProcessor processor)
{
PdfLiteral operatorLit = new PdfLiteral("J");
List<PdfObject> operands = new List<PdfObject> { new PdfNumber(PdfContentByte.LINE_CAP_ROUND), operatorLit };
base.Write(processor, operatorLit, operands);
operatorLit = new PdfLiteral("j");
operands = new List<PdfObject> { new PdfNumber(PdfContentByte.LINE_JOIN_ROUND), operatorLit };
base.Write(processor, operatorLit, operands);
}
List<string> CAP_AND_JOIN_OPERATORS = new List<string> { "j", "J" };
string GSTATE_OPERATOR = "gs";
bool start = true;
}
Applying it like this to the PDF above
using (PdfReader pdfReader = new PdfReader(testDocument))
using (PdfStamper pdfStamper = new PdfStamper(pdfReader, new FileStream(@"Paths-Rounded.pdf", FileMode.Create, FileAccess.Write), (char)0, true))
{
pdfStamper.RotateContents = false;
PdfContentStreamEditor editor = new PathMakeCapAndJoinRound();
for (int i = 1; i <= pdfReader.NumberOfPages; i++)
{
editor.EditPage(pdfStamper, i);
}
}
we get the result:

Beware, the restrictions from the referenced answer remain. In particular this editor only edits the page content stream. For a complete solution you have to also edit all form XObject and Pattern streams, and also deal with annotations.
To allow reproduction, this is how I created my test document:
byte[] createMixedPathsPdf()
{
using (MemoryStream memoryStream = new MemoryStream())
{
using (Document document = new Document())
{
PdfWriter writer = PdfWriter.GetInstance(document, memoryStream);
document.Open();
var canvas = writer.DirectContent;
canvas.SetLineWidth(10);
canvas.MoveTo(100, 700);
canvas.CurveTo(180, 720, 180, 720, 200, 800);
canvas.CurveTo(220, 720, 220, 720, 350, 700);
canvas.MoveTo(350, 700);
canvas.CurveTo(220, 680, 220, 680, 210, 650);
canvas.Stroke();
canvas.SetLineCap(PdfContentByte.LINE_CAP_BUTT);
canvas.SetLineJoin(PdfContentByte.LINE_JOIN_BEVEL);
canvas.SetGState(createGState(PdfContentByte.LINE_CAP_BUTT, PdfContentByte.LINE_JOIN_BEVEL));
canvas.MoveTo(100, 500);
canvas.CurveTo(180, 520, 180, 520, 200, 600);
canvas.CurveTo(220, 520, 220, 520, 350, 500);
canvas.MoveTo(350, 500);
canvas.CurveTo(220, 480, 220, 480, 210, 450);
canvas.Stroke();
canvas.SetLineCap(PdfContentByte.LINE_CAP_PROJECTING_SQUARE);
canvas.SetLineJoin(PdfContentByte.LINE_JOIN_MITER);
canvas.SetGState(createGState(PdfContentByte.LINE_CAP_PROJECTING_SQUARE, PdfContentByte.LINE_JOIN_MITER));
canvas.MoveTo(100, 300);
canvas.CurveTo(180, 320, 180, 320, 200, 400);
canvas.CurveTo(220, 320, 220, 320, 350, 300);
canvas.MoveTo(350, 300);
canvas.CurveTo(220, 280, 220, 280, 210, 250);
canvas.Stroke();
canvas.SetLineCap(PdfContentByte.LINE_CAP_ROUND);
canvas.SetLineJoin(PdfContentByte.LINE_JOIN_ROUND);
canvas.SetGState(createGState(PdfContentByte.LINE_CAP_ROUND, PdfContentByte.LINE_JOIN_ROUND));
canvas.MoveTo(100, 100);
canvas.CurveTo(180, 120, 180, 120, 200, 200);
canvas.CurveTo(220, 120, 220, 120, 350, 100);
canvas.MoveTo(350, 100);
canvas.CurveTo(220, 080, 220, 080, 210, 050);
canvas.Stroke();
}
return memoryStream.ToArray();
}
}
PdfGState createGState(int lineCap, int lineJoin)
{
PdfGState pdfGState = new PdfGState();
pdfGState.Put(new PdfName("LC"), new PdfNumber(lineCap));
pdfGState.Put(new PdfName("LJ"), new PdfNumber(lineJoin));
return pdfGState;
}