One way to do this would be to simply loop from the first date to the second date, adding an hour on each iteration, and then incrementing a variable depending on the value of the Hour
:
private static void Main()
{
DateTime date1 = new DateTime(2019, 2, 20, 16, 0, 0);
DateTime date2 = new DateTime(2019, 2, 20, 23, 0, 0);
var hoursInFirstRange = 0;
var hoursInSecondRange = 0;
// If you want to include the final hour (23) change '<' to '<=' below
for (DateTime temp = date1; temp < date2; temp = temp.AddHours(1))
{
if (temp.Hour >= 8 && temp.Hour <= 20) hoursInFirstRange++;
else hoursInSecondRange++;
}
Console.WriteLine($"From {date1} to {date2} there are:");
Console.WriteLine($" - {hoursInFirstRange} hours between 8:00 and 20:00");
Console.WriteLine($" - {hoursInSecondRange} hours between 20:00 and 8:00");
GetKeyFromUser("\nDone! Press any key to exit...");
}
Output
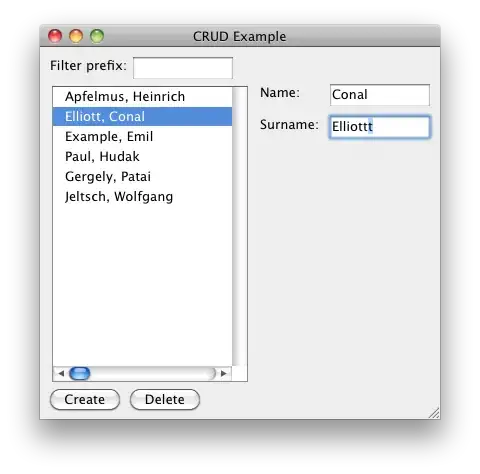