You can achieve this by using a custom script and having it run before the build executes. Android seems to ignore anything in layout subdirectories, so you can safely put your files into them. The following ruby script (written for Linux, but easily convertible to other platforms) will then delete everything that's not a directory in res/layout/ and copy every file from the subdirs into res/layout/:
#!/usr/bin/ruby
require "fileutils"
def collect_files(directory)
FileUtils.cd(directory)
FileUtils.rm(Dir.entries(directory).reject{|x| File.directory?(x)}) #Remove all layout files in base dir
files_to_copy=Dir.glob("**/*").reject{|x| File.directory?(x)}
files_to_copy.each{|x| print "Copying #{x} to #{directory}\n"}
FileUtils.cp(files_to_copy, directory) #Copy all files in subdir into base dir
end
if ARGV[0]!=nil && File.directory?(ARGV[0])
xml_dir=ARGV[0]
layout_dir="#{xml_dir}/layout"
collect_files(layout_dir)
else
puts("Must specify a valid directory!")
end
Be warned that the above script is not robust, and will actually delete any layout files not in a subdirectory. You can always remove the deletion step if you like, but then any files you remove from the subdirectories will remain in the main directory for subsequent builds.
If you're running Eclipse, you can then configure an external tool, which you can add to your builders later. Just open up Run -> External Tools -> External Tools Configurations, and create a new tool under 'Programs'. Here a screenie of my settings:
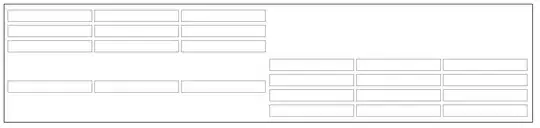
Note: The working directory is a red herring, and won't be used. You'll need to specify the location where you drop the script, not the one shown here
Now you can add the tool to the builders for your project. Select your project and open up Project -> Properties. Now Select the 'Builders' item and click 'Import'. You should see your tool there if you defined it successfully. It needs to run before the rest of the build process, so make sure to move it up to the top of the list. Here's what it should look like when you're done:

Now you just move layout files into subdirectories (but watch out for name collisions, remember the files will all end up in the same directory for the build!) and build your project. You'll see them magically appear in the root of /res/layout/ when you do this and your app should then build normally.
Caveat Scriptor: If you're specifying multiple layouts, or anything else which uses more than just the /res/layout/ directory, you'll need to extend this script or add the tool multiple times for the different directories to handle it. I don't personally use this technique, and so haven't seen where it falls down, but have performed a test with a basic android Hello World app with a couple of layouts in some subdirectories.
Also, my script will break if used with paths containing spaces!