First of all, trim dollar ($
) character from your input string and then split it by comma (,
),
string str = "$value1,value2,value3,value4,value5$";
string[] values = str.Trim('$').Split(','); //<= You can add ".ToArray()" at the end for your understaning.
Output: (From Debugger)
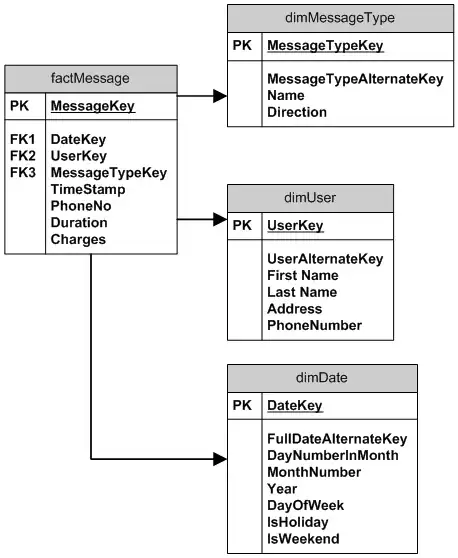
Then you can easily get each of your string in above array to separate string variable,
string value1 = values[0];
string value2 = values[1];
string value3 = values[2];
string value4 = values[3];
string value5 = values[4];
If your values are undetermined then you can simply use foreach loop to access each value like,
foreach(string value in values)
{
//Do code with each value
}