Simple examples for a 1-D array
array to row:
Sub arrayTest()
Dim arr(1 To 3) As String
Dim r1 As Range
Set r1 = Range("A1:C1")
arr(1) = "larry"
arr(2) = "moe"
arr(3) = "curly"
r1 = arr
End Sub
or array to column:
Sub arrayTest2()
Dim arr(1 To 3) As String
Dim r1 As Range
Set r1 = Range("A1:A3")
arr(1) = "larry"
arr(2) = "moe"
arr(3) = "curly"
r1 = Application.WorksheetFunction.Transpose(arr)
End Sub
A 2-D array is equally easy:
Sub arrayTest3()
Dim arr(1 To 3, 1 To 2) As String
Dim r1 As Range
Set r1 = Range("A1:B3")
arr(1, 1) = "larry"
arr(2, 1) = "moe"
arr(3, 1) = "curly"
arr(1, 2) = "gary"
arr(2, 2) = "student"
arr(3, 2) = "whatever"
r1 = arr
End Sub
EDIT#1:
Say we have an arbitrary 1-D array, either zero-based or one-based, and we want to push it into a row starting with cell B9:
Sub arrayTest4()
Dim r1 As Range, U As Long, L As Long, rBase As Range
Set rBase = Range("B9")
arr = Array("qwert", 1, 2, 3, 4, "ytrew", "mjiop", "nhy789")
L = LBound(arr)
U = UBound(arr)
Set r1 = rBase.Resize(1, U - L + 1)
r1 = arr
End Sub
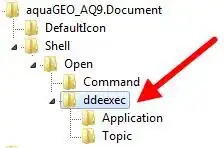