My answer to our expanded requirements
I wanted to document our approach for others that are trying to deal with one or both of the issues that I noted in the original question.
As others have already pointed out, you cannot achieve intermediate font-weights using CSS text-shadow. If you tried to emulate font-weights with JavaScript and CSS (really bad idea), it would be convolutedly complex and you would never achieve the good cross-platform uniformity that the font-weight functionality provides.
Side Note: Notice the Stack Overflow font bolding of the word JavaScript. The font-width and height is noticeably bigger in Firefox, which is what I am using now. This is a normal side effect of changing font-weight for inline text.
Problem 1. Missing Font Weight (less bold increments)
The following illustrates one of two problems we have been trying to solve. The first example uses web safe fonts. The second uses Open-Sans to achieve the desired half-tone font-weight. This solved our first problem. No, I am not shouting. There's just no way to add a subtle text effect on SO, and none is required. Here is a good link with font images. This document lead me to make the choice of the Open Sans font.
The first bold font is the only choice with the web safe font that we were using. The second is the Open Sans font that we downloaded from Google. The pasted images do not do either font justice. If you run the code snippet, you will see what it really looks like.
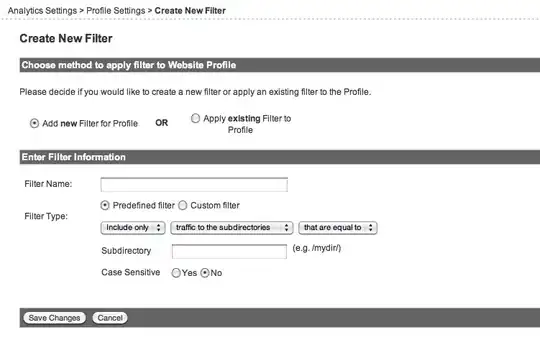
From the above, you can observe how the bolder font-weight affects the text using the web safe Arial font-family. The Open Sans font is more like what we were looking for. However, if you want to add inline emphasis to a word or set of words, the switch between the 500 and 600 font-weight causes a noticeable increase in font height and thickening of the font. This, in MHO, is not the best solution from an aesthetic perspective.
Problem 2. Subtle Text Accents (less bold than next font-weight that do not change text width or height)
First, compare the more subtle versions (below) of the above two tables using text-shadowing effects.
If you run the snippet and use the + key to zoom in one or two levels, the text accent is still discernible.
The tables below are a little smaller because the text shadowing does not affect the element width or height (see W3.org note below). Text-shadows can infringe on neighboring characters, which can be bad or good, depending on the designers intent. BTW, our users picked the second set of highlighted table fonts 100% of the time. They said they looked clearer, cleaner, sharper, not so thick, etc.
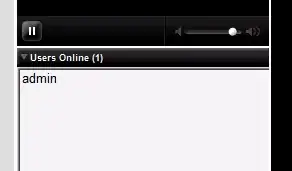
From W3.org:
- Unlike box-shadow, text shadows are not clipped to the shadowed shape and may show through if the text is partially-transparent.
- Like box-shadow, text shadows do not influence layout, and do not trigger scrolling or increase the size of the scrollable area.
Have a look at the paragraph below. It is included in the code snippet. It is pretty easy to pick out one set of highlighted words that are thicker and a little higher than adjacent text. If you run the snippet, you'll notice the fonts are clearer than the reproduced image below. Our users vastly preferred the more subtle text highlighting for inline text and tables.
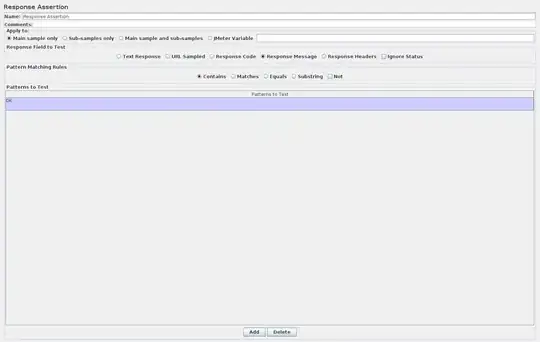
We have always used a darker text color for words that we wanted to accent. For larger fonts, our users said that it was 'adequate', but 'not great'. Their complaint was that the color difference was not really visible for smaller font sizes.
The problem is that black #000 fonts turn into shades of lighter grays (see next figure below) as the font size is made smaller, which is a font-rendering issue that we cannot change. This is our second issue.
We were able to address this, to our satisfaction, when we added a small/horizontal sub-pixel text shadow. We were able to achieve a comparatively clear, bolder looking text accent that can be observed even as the font size is increased (say 14px to 28px). Our users were stoked, to say the least, with the visual improvements of the new font and text shadow highlighting effects.
You can judge for yourself by running the example on different OS, devices, and browsers. We did just that with our users. We let them determine their preference with side-by-side comparisons of devices (mobile/PC) on the same and different operating systems.
Caveats
- Text shadowing requires some experimentation. Text-weights just work.
- All browsers, OSs, and hardware text rendering are not created. Expect variability.
- Expect the text highlighting effect to diminish when the font size is increases.
- If you can use the darkest color hue for the text accents, the difference in color hues can still be seen at much larger fonts, which provide a path to graceful degradation.
The following 12 lines of text are divided into two groups.
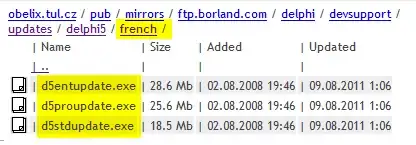
The first group of 6 lines reflect the default Open Sans 600 font in the left column and accented text applied to the 600 font weight in the right column.
The second group of 6 lines reflect the default Open Sans 500 font in the left column and accented text applied to the 500 font weight in the right column.
Again, if you run the code snippet, you'll see better quality results over the copy/paste.
The Stack Overflow question, here, also addresses our second issue. The solution is the simplest one I have found, but it gives blurry result at more than ~0.3px for text-shadow: 0 0 0.3px;
<div>Testing 123: </div>
<div style="text-shadow: 0 0 .2px">Testing 123: </div>
<div style="text-shadow: 0 0 .4px">Testing 123: </div>
<div style="text-shadow: 0 0 .6px">Testing 123: </div>
<div style="text-shadow: 0 0 .8px">Testing 123: </div>
<div style="text-shadow: 0 0 1px">Testing 123: </div>
@import url('https://fonts.googleapis.com/css?family=Open+Sans:400,600');
body {
padding: 0;
margin: 0;
font-family: 'Open Sans', sans-serif;
font-weight: normal;
font-size: 15px;
font-style: normal;
font-variant: normal;
text-transform: none;
font-synthesis: none;
-webkit-font-smoothing: antialiased;
-webkit-font-smoothing: subpixel-antialiased;
-moz-osx-font-smoothing: grayscale;
color: #383838;
width: 100%;
height: 100%;
-webkit-text-size-adjust: none !important;
-ms-text-size-adjust: none !important;
-moz-text-size-adjust: none !important;
border: none;
text-align: center;
text-rendering: optimizelegibility;
min-width: 300px !important;
}
h3,
h4,
h5,
h6 {
display: block;
font-family: inherit;
color: rgba(0, 0, 0, .83);
text-align: center;
margin: 10px auto 6px auto;
font-size: 1em;
font-weight: 500;
}
h3 {
font-weight: 500;
font-size: 1em;
color: rgba(0, 0, 0, 0.9);
text-shadow: 0.45px 0 0 rgba(0, 0, 0, 0.9);
}
h4 {
color: rgba(0, 0, 0, 0.9);
text-shadow: 0.1px 0 0 rgba(0, 0, 0, 0.9);
text-shadow: 0.3px 0 0 rgba(0, 0, 0, 0.9);
font-size: 1em;
}
h5,
.darker-font {
color: rgba(0, 0, 0, 0.9);
text-shadow: 0.08px 0 0 rgba(0, 0, 0, 0.9);
font-size: 1em;
}
.center-block {
display: block;
width: auto;
text-align: center;
margin-right: auto;
margin-left: auto;
}
.margintb-8 {
margin-top: 8px;
margin-bottom: 8px;
}
.column0,
.column1,
.column2,
.column3,
.column-w {
display: table-column;
width: auto;
align-content: center;
}
.table-r {
display: table;
border: 1px solid #606060;
margin: auto;
align-content: center;
background: #fff;
}
.row-r {
display: table-row;
}
.cell-r-center,
.cell-r-left,
.cell-r-right,
.cell-r-center-border,
.cell-r-left-border,
.cell-r-right-border {
display: table-cell;
text-align: center;
padding: 4px;
white-space: nowrap;
}
.cell-r-left,
.cell-r-left-border {
text-align: left;
}
.cell-r-right,
.cell-r-right-border {
text-align: right;
}
.cell-r-center-border,
.cell-r-left-border,
.cell-r-right-border {
border-top: 1px solid #606060;
}
div {
font-family: inherit;
color: #333;
text-align: left;
}
.inline-block {
vertical-align: middle;
display: inline-block;
}
.shadow-text-bold,
.shadow-text-bolder,
.shadow-text-boldest {
font-weight: 500;
color: rgba(0, 0, 0, 0.84);
text-shadow: 0.15px 0.15px 0.1px rgba(0, 0, 0, 0.84)
}
.shadow-text-bolder {
text-shadow: 0.3px 0.3px 0.1px rgba(0, 0, 0, 0.84)
}
.shadow-text-boldest {
color: rgba(0, 0, 0, 0.9);
text-shadow: 0.08px 0 0 rgba(0, 0, 0, 0.9);
}
<!DOCTYPE html>
<head></head>
<html>
<body>
<br />
<div>The first two tables apply default font-weights of 500 and 600 to achieve text highlighting betweeen column headings and table content.</div>
<div class="center-block">
<div style="font-family: Arial, Helvetica, sans-serif;">
<div class="center-block margintb-8" style="font-weight: 600; color: #000">Subscriptions Arial Web Safe</div>
<div class="center-block">
<div class="text-container-light-overflow-container">
<div class="table-r">
<div class='column0'></div>
<div class='column-w'></div>
<div class='column-w'></div>
<div class='row-r'>
<div class='cell-r-center' style="font-weight: 600">#</div>
<div class='cell-r-center' style="font-weight: 600">Expiration</div>
<div class='cell-r-center' style="font-weight: 600">Available</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border cell-40'> 1 </div>
<div class='cell-r-center-border cell-100'>07/01/2027</div>
<div class='cell-r-center-border cell-100'> $1927.90</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border cell-40'> 2 </div>
<div class='cell-r-center-border cell-100'>07/01/2027</div>
<div class='cell-r-center-border cell-100'> $1400.00</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border'> </div>
<div class='cell-r-center-border'>--------</div>
<div class='cell-r-center-border' style="font-weight: 600"> $2327.90</div>
</div>
</div>
</div>
</div>
</div>
<div style="font-family: 'Open Sans';">
<div class="center-block margintb-8" style="font-weight: 600; color: #000">Subscriptions Open Sans</div>
<div class="center-block">
<div class="text-container-light-overflow-container">
<div class="table-r">
<div class='column0'></div>
<div class='column-w'></div>
<div class='column-w'></div>
<div class='row-r'>
<div class='cell-r-center' style="font-weight: 600">#</div>
<div class='cell-r-center' style="font-weight: 600">Expiration</div>
<div class='cell-r-center' style="font-weight: 600">Available</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border cell-40'> 1 </div>
<div class='cell-r-center-border cell-100'>07/01/2027</div>
<div class='cell-r-center-border cell-100'> $1927.90</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border cell-40'> 2 </div>
<div class='cell-r-center-border cell-100'>07/01/2027</div>
<div class='cell-r-center-border cell-100'> $1400.00</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border'> </div>
<div class='cell-r-center-border'>--------</div>
<div class='cell-r-center-border' style="font-weight: 600"> $2327.90</div>
</div>
</div>
</div>
</div>
</div>
<br />
<div style="font-family: 'Open Sans';">
<span>The</span>
<span class="shadow-text-boldest">second two tables</span><span> apply default font-weight of 500 and subtle text-shadowing to achieve text highlighting betweeen column headings and table content. There are also</span>
<span class="shadow-text-boldest">three examples</span><span> of inline text highlighting in this paragraph to enhance content without screaming or altering the base text-font width, which is a <span class="shadow-text-boldest">real plus at times</span>.
Just remember that the text-shadow effect will diminish as the font-size is increased and can cause <span style="font-weight: 600">unwanted destortions</span> when the font-size is made <span class="shadow-text-boldest" style="font-size: 6px; font-weight: 600;">very small.</span></span>.
Notice the second from last "unwanted destortions" text. It is the Open Sans font at 600 font-weight. It is noticeably higher and thicker than the adjacent text. Compare to the text-shadow versions earlier in the paragraph.
</div>
<div style="font-family: Arial, Helvetica, sans-serif;">
<div class="center-block shadow-text-bold margintb-8">Subscriptions Arial Web Safe</div>
<div class="center-block">
<div class="text-container-light-overflow-container">
<div class="table-r">
<div class='column0'></div>
<div class='column-w'></div>
<div class='column-w'></div>
<div class='row-r'>
<div class='cell-r-center shadow-text-bold'>#</div>
<div class='cell-r-center shadow-text-bold'>Expiration</div>
<div class='cell-r-center shadow-text-bold'>Available</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border cell-40'> 1 </div>
<div class='cell-r-center-border cell-100'>07/01/2027</div>
<div class='cell-r-center-border cell-100'> $1927.90</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border cell-40'> 2 </div>
<div class='cell-r-center-border cell-100'>07/01/2027</div>
<div class='cell-r-center-border cell-100'> $1400.00</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border'> </div>
<div class='cell-r-center-border'>--------</div>
<div class='cell-r-center-border shadow-text-bold'> $2327.90</div>
</div>
</div>
</div>
</div>
</div>
<div style="font-family: 'Open Sans';">
<div class="center-block shadow-text-bold margintb-8">Subscriptions Open Sans</div>
<div class="center-block">
<div class="text-container-light-overflow-container">
<div class="table-r">
<div class='column0'></div>
<div class='column-w'></div>
<div class='column-w'></div>
<div class='row-r'>
<div class='cell-r-center shadow-text-bold'>#</div>
<div class='cell-r-center shadow-text-bold'>Expiration</div>
<div class='cell-r-center shadow-text-bold'>Available</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border cell-40'> 1 </div>
<div class='cell-r-center-border cell-100'>07/01/2027</div>
<div class='cell-r-center-border cell-100'> $1927.90</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border cell-40'> 2 </div>
<div class='cell-r-center-border cell-100'>07/01/2027</div>
<div class='cell-r-center-border cell-100'> $1400.00</div>
</div>
<div class='row-r'>
<div class='cell-r-center-border'> </div>
<div class='cell-r-center-border'>--------</div>
<div class='cell-r-center-border shadow-text-bold'> $2327.90</div>
</div>
</div>
</div>
</div>
<div class="center-block">
<div class="center-block margint-16" style="font-weight: 600; color: rgba(0, 0, 0, 0.9);">
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0.65px 0 0 rgba(0, 0, 0, 0.9)">Testing 123</span>
</div>
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0.55px 0 0 rgba(0, 0, 0, 0.9)">Testing 123</span>
</div>
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0.45px 0 0 rgba(0, 0, 0, 0.9)">Testing 123</span>
</div>
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0.3px 0 0 rgba(0, 0, 0, 0.9)">Testing 123</span>
</div>
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0.08px 0 0 rgba(0, 0, 0, 0.9)">Testing 123</span>
</div>
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0 0">Testing 123</span>
</div>
</div>
<div class="center-block" style="color: rgba(0, 0, 0, 0.9)">
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0.65px 0 0 rgba(0, 0, 0, 0.9)">Testing 123</span>
</div>
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0.55px 0 0 rgba(0, 0, 0, 0.9)">Testing 123</span>
</div>
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0.45px 0 0 rgba(0, 0, 0, 0.9)">Testing 123</span>
</div>
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0.3px 0 0 rgba(0, 0, 0, 0.9)">Testing 123</span>
</div>
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0.08px 0 0 rgba(0, 0, 0, 0.9)">Testing 123</span>
</div>
<div>
<span class="inline-block">Testing 123: </span><span class="inline-block" style="text-shadow: 0 0 rgba(0, 0, 0, 0.0);">Testing 123</span>
</div>
</div>
</div>
</div>
</div>
</body>
</html>