SensorEvent.timestamp
is when the event happened, but it is an elapsed time since boot. It is not the time when the event happened. We want to get the actual time when the event happened from the elapsed time since the event happened.
Reference: https://developer.android.com/reference/android/hardware/SensorEvent#timestamp
- We need all the entities in a similar unit. We are taking
Millis
here.
- We have three entities to use. a)
System.currentTimeMillis()
b) SystemClock.elapsedRealtime()
and c) SensorEvent.timestamp
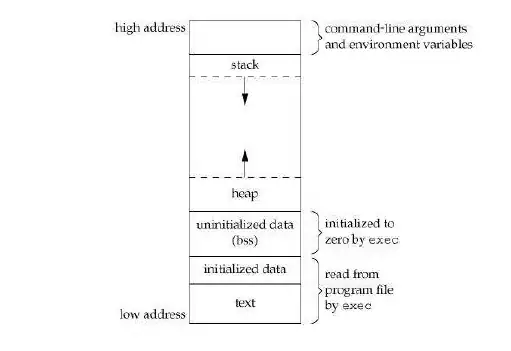
- If we use
SystemClock.elapsedRealtimeNanos()
, then we need to convert it into Millis
as below:
val systemCurrentTimeMillis = System.currentTimeMillis()
val systemClockElapsedRealtimeMillis = TimeUnit.NANOSECONDS.toMillis(SystemClock.elapsedRealtimeNanos())
val sensorEventTimeStampMillis = TimeUnit.NANOSECONDS.toMillis(sensorEvent.timestamp)
- We need to find the difference between the
systemCurrentTimeMillis
, and systemClockElapsedRealtimeMillis
as below:

val currentMinusElapsedRealtimeMillis = systemCurrentTimeMillis - systemClockElapsedRealtimeMillis
- Once we find the difference, we need to add
sensorEventTimeStampMillis
to it as below:
OR

val actualEventTimeMillis = currentMinusElapsedRealtimeMillis + sensorEventTimeStampMillis
- Then, we need to convert the result into UTC as below (I am using
Joda Time
):
val actualEventTimeUtc = DateTime(actualEventTimeMillis, DateTimeZone.UTC)
The actualEventTimeUtc
is the absolute time when the event happened.
Another way to understand it is:
Suppose the sensor reports an event in onSensorChanged.
We find that the current time is: 13 PM. In other words, the reporting time is 13 PM and it is not the time when the actual event happened.
The SystemClock.elapsedRealtime()
says that it has been running since last 30 hours. In other words, it started 30 hours before from the current time.
So, we subtract the 30 hours from the current time to get the time when the sensor started. So, we get 7 AM of the previous day.
The SensorEvent.timestamp
says that the event happened after 28 hours of the SystemClock.elapsedRealtime().
Hence, we add 28 hours to the 7 AM of the previous day. So, we get 11 AM of the current day and it is the actual time when event happened.