I used String.split(String regex) method and see the Array has 5 elements. I hope this answers your query.
package net.javapedia.StringSplitExample;
public class Main {
public static void main (String[] s) {
String s1 = "a, b, c, d, e";
String s2 = "a, , , d, ";
String s3 = "a, b, c, d, ";
// This is the String that issue is reported
String s4 = "a,b,c,d,";
String s5 = "a,b,c,d,";
String[] s1Array= s1.split(",");
String[] s2Array= s2.split(",");
String[] s3Array= s3.split(",");
//Below split ignore empty string
String[] s4Array= s4.split(",");
//Below split doesn't
String[] s5Array= s5.split(",",-1);
System.out.println(s1Array.length);
System.out.println(s2Array.length);
System.out.println(s3Array.length);
//Prints 4
System.out.println(s4Array.length);
//Prints 5 :)
System.out.println(s5Array.length);
}
}
Output:
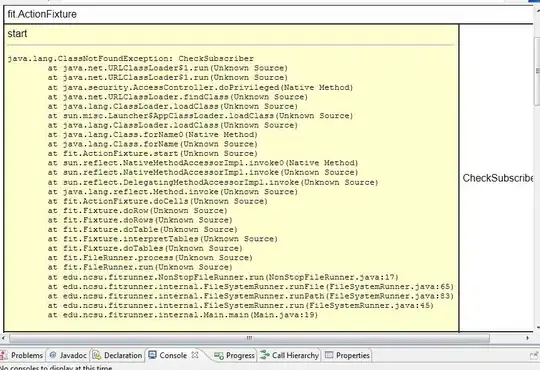
Reference