Create style.css file as below and drag & drop to your project directory
@font-face {
font-family: "Titillium Web";
font-weight: normal;
src: url("TitilliumWeb-Regular.ttf")
}
@font-face {
font-family: "Titillium Web";
font-style: italic;
src: url("TitilliumWeb-Italic.ttf")
}
@font-face {
font-family: "Titillium Web";
font-weight: bold;
src: url("TitilliumWeb-Bold.ttf")
}
body {
margin: 0;
font-family: "Titillium Web";
font-weight: normal;
font-size: 13pt;
}
Add below source code in UIViewController's viewDidLoad()
override func viewDidLoad() {
super.viewDidLoad()
let html = """
"Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. <b>Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.</b>
<br>
<i>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</i>
<br>
Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur."
"""
let htmlStart = "<HTML><HEAD><meta name=\"viewport\" content=\"width=device-width, initial-scale=1.0, shrink-to-fit=no\"></HEAD><BODY>"
let htmlEnd = "</BODY></HTML>"
let htmlString = "\(htmlStart)\(html)\(htmlEnd)"
webView.loadHTMLString(htmlString, baseURL: Bundle.main.bundleURL)
self.view.addSubview(webView)
}
Create WKWebView object as below and run the app, It will load the content.
lazy var webView: WKWebView = {
guard
let path = Bundle.main.path(forResource: "style", ofType: "css"),
let cssString = try? String(contentsOfFile: path).components(separatedBy: .newlines).joined()
else {
return WKWebView()
}
let source = """
var style = document.createElement('style');
style.innerHTML = '\(cssString)';
document.head.appendChild(style);
"""
let userScript = WKUserScript(source: source,
injectionTime: .atDocumentEnd,
forMainFrameOnly: true)
let userContentController = WKUserContentController()
userContentController.addUserScript(userScript)
let configuration = WKWebViewConfiguration()
configuration.userContentController = userContentController
let webView = WKWebView(frame: self.view.frame,
configuration: configuration)
return webView
}()
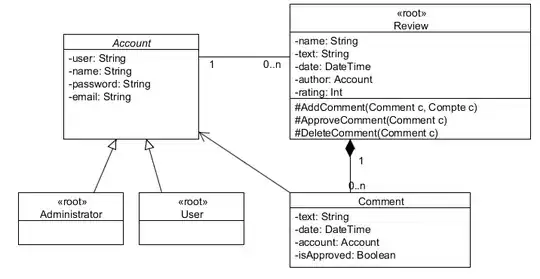