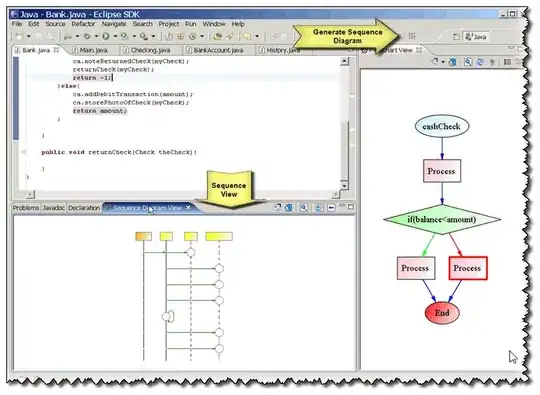
This solution will detect if your Image
has been fully visible on your user screen, and will change the AppBar
title if so. Assuming you want to display a single page with some content and an Image
:
class ImageDisplayDetection extends StatefulWidget {
ImageDisplayDetection({Key key,}) : super(key: key);
@override
_ImageDisplayDetectionState createState() => _ImageDisplayDetectionState();
}
class _ImageDisplayDetectionState extends State<ImageDisplayDetection> {
ScrollController _controller; // To get the current scroll offset
var _itemSize = 400.0 ; // The height of your image
double _listSize = 2000.0 ;
double position = 1500.0 ; // position from the top of the list where the image begins
var seen = false ; // to report the visibility of your image
@override
void initState() {
_controller = ScrollController();
_controller.addListener(_scrollListener); // The listener will be used to check if the image has become visible
super.initState();
}
_scrollListener() {
setState(() {
// This 60.0 is the assumed hieght of the bottom navigation buttons so the image won't be considered visible unless it is above these buttons
if((_controller.offset + MediaQuery.of(context).size.height) >= position + _itemSize + 60.0){
seen = true ;
}
});
}
@override
Widget build(BuildContext context) {
return new Scaffold(
backgroundColor: Colors.grey.shade200 ,
appBar: new AppBar(title: new Text(seen ? 'Image Displayed Successfully' : 'Image not displayed'),),
body: ListView.builder(
controller: _controller ,
itemCount: 1,
itemBuilder: (context, index) {
return Container(
height: _listSize ,
child: new Stack(
children: <Widget>[
// You can include other childern here such as TextArea
Positioned(
top: position,
child: SizedBox(
height: _itemSize,
width: _itemSize,
child: ClipRRect(
borderRadius: BorderRadius.circular(5.0),
child: Image.asset('assets/images/2.jpg'), //Change the image widget to match your own image source and name
),
),
),
],
),
);
}),
);
}
}
If you rather want a listview
with multiple ListTiles
you can opt for this answer which can detect whether a child
which an arbitrary index has become visible and is displayed at certain position in the screen.