The solution that you are looking for is little different than what someone expect. JavaScript's JSON.stringify()
generates JSON
string and a valid JSON
contains "
(double quotes only) around keys.
In your case, you are trying to use the JSON string without "
around keys. So here is a little simple process to do that. Here I am assuming that you are going to use this in simple kind of JSON
strings where the value part of any key don't have key:
kind of things then it will work fine of bigger JSONs
too.
If it is not like that then you will need to improve the find & replace utility in more efficient form. Regular expressions are great for this work.
Here I have tried to solve your problem like this.
I have used NODE REPL to execute statements so please ignore undefined
returned by default.
>
> let o = {
... "name": "Test Name",
... "age": 24
... }
undefined
>
> s = JSON.stringify(o)
'{"name":"Test Name","age":24}'
>
> s = JSON.stringify(o, undefined, 4)
'{\n "name": "Test Name",\n "age": 24\n}'
>
> console.log(s)
{
"name": "Test Name",
"age": 24
}
undefined
>
> for(k in o) {
... s = s.replace("\"" + k + "\":", k + ':')
... }
'{\n name: "Test Name",\n age: 24\n}'
>
> console.log(s)
{
name: "Test Name",
age: 24
}
undefined
>
you can have a look at this as well.
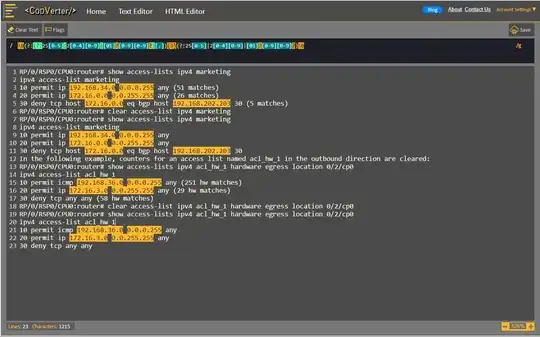