The referenced file has an overly ornate header which must be treated. Presuming you are receiving this file repeatedly, from a source that cannot be altered, such as a server or experiment apparatus, you are left to programmatically handle the following:
MTS793|MPT|ENU|1|2|.|/|:|1|0|0|A
Operator Information Time: 15.133789 Sec 8/2/2018 12:18:30 PM
Is the extensometer pin pulled? ughiopuh;u
Operator Information End
Data Acquisition Tensile Test Data Time: 117.81934 Sec 8/2/2018 12:20:13 PM
Time Axial Displacement Axial Strain Axial Force
Sec in in/in lbf
This must be removed to a proper set of headers to successfully use pandas to import the file.
Time;Axial Displacement;Axial Strain;Axial Force
Sec in;in/in;lbf
are likely the headers desired. This gets you into column multiindex world which is getting advanced.
Here is a functioning answer:
import openpyxl
import pandas as pd
import sys
if sys.version_info[0] < 3:
from StringIO import StringIO
else:
from io import StringIO
csvdata = StringIO("""MTS793|MPT|ENU|1|2|.|/|:|1|0|0|A
Operator Information Time: 15.133789 Sec 8/2/2018 12:18:30 PM
Is the extensometer pin pulled? ughiopuh;u
Operator Information End
Data Acquisition Tensile Test Data Time: 117.81934 Sec 8/2/2018 12:20:13 PM
Time Axial Displacement Axial Strain Axial Force
Sec in in/in lbf
15.372559 -0.00026854035 -0.00013428145 -3.7030973
15.472656 2.2532094e-08 -8.3925901e-05 11.109222
15.572754 -0.00026854035 -0.00011749626 3.7030623
15.672852 2.2532094e-08 -0.00011749626 -1.7583034e-05
15.772949 0.00026858543 -0.00010071108 7.4061422""")
df = pd.read_csv(csvdata, sep="\t", header=[5,6])
# Show how pandas parses the file headers
print(df.head(6))
# The columns are referenceable as a multiindex
level0 = df.columns.get_level_values(0)
level1 = df.columns.get_level_values(1)
# Set index from multiindex columns!
# But we are not going to assign this result, because
# there is a better trick
print(df.set_index(('Time', 'Sec')))
flattened_cols = (["{} ({})".format(x,y) for x,y in zip(level0, level1)])
df.columns = flattened_cols
# The better trick
df.set_index(flattened_cols[0], inplace=True)
# show results
print(df.head(6))
# save in desired file format
df.to_excel('specimen.xlsx')
The result in memory
Axial Displacement (in) Axial Strain (in/in) Axial Force (lbf)
Time (Sec)
15.372559 -2.685404e-04 -0.000134 -3.703097
15.472656 2.253209e-08 -0.000084 11.109222
15.572754 -2.685404e-04 -0.000117 3.703062
15.672852 2.253209e-08 -0.000117 -0.000018
15.772949 2.685854e-04 -0.000101 7.406142
The result in xls
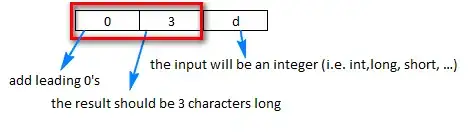
I deserve a straight up 'accepted answer' for this one! Best of luck.