You can achieve that using:
ConstraintLayout
ConstraintLayout allows you to create complex layouts with a flat view hierarchy (no nested view groups, like LinearLayout
in your example). It's similar to RelativeLayout
in that all views are laid out according to relationships between sibling. ConstraintLayout
has much more flexibility and performs better than RelativeLayout
.
ConstraintLayout
is available as a support library so you have to add new dependency:
implementation 'com.android.support.constraint:constraint-layout:1.1.3'
You can find posts about differences between ConstraintLayout and RelativeLayout or How to use constraint (relations)
Example:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical"
android:padding="30dp"
tools:context=".MainActivity">
<ImageView
android:id="@+id/first_logo"
android:layout_width="30dp"
android:layout_height="30dp"
android:background="#F00"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/first_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="JUVENTUS"
app:layout_constraintBottom_toBottomOf="@id/first_logo"
app:layout_constraintStart_toEndOf="@id/first_logo"
app:layout_constraintTop_toTopOf="@id/first_logo" />
<TextView
android:id="@+id/second_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="end"
android:text="REAL MADRID"
app:layout_constraintBottom_toBottomOf="@id/second_logo"
app:layout_constraintEnd_toStartOf="@id/second_logo"
app:layout_constraintTop_toTopOf="@id/second_logo" />
<ImageView
android:id="@+id/second_logo"
android:layout_width="30dp"
android:layout_height="30dp"
android:layout_gravity="end"
android:background="#00F"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>

LinearLayout
LinearLayout
is a view group that aligns all children in a single direction (vertically or horizontally like in your example). You can set android:layout_weight
on individual child views to specify how linear layout divides remaining space amongst the views it contains.
There are many posts about that:
Example:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="16dp"
android:paddingRight="16dp">
<ImageView
android:layout_width="30dp"
android:layout_height="30dp"
android:background="#F00" />
<TextView
android:layout_width="0dp"
android:layout_height="30dp"
android:layout_weight="1"
android:gravity="center_vertical"
android:text="JUVENTUS" />
<TextView
android:layout_width="0dp"
android:layout_height="30dp"
android:layout_gravity="end"
android:layout_weight="1"
android:gravity="center_vertical|end"
android:text="REAL MADRID" />
<ImageView
android:layout_width="30dp"
android:layout_height="30dp"
android:background="#00F" />
</LinearLayout>
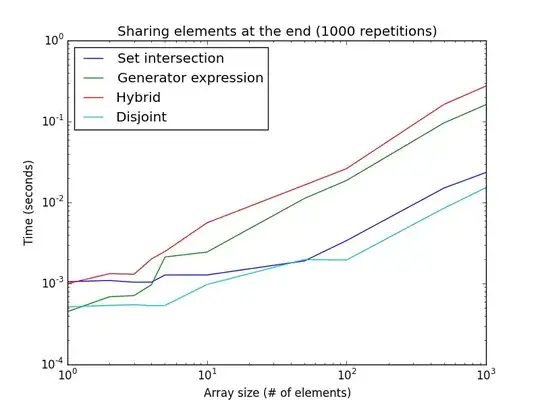