It seems that you need to get avg
based on columns index instead of rows then .Zip
will be one option for you,
Suppose your list of list of string is,
var myList = new List<List<string>>
{
new List<string> { "1.11, 2.11, 3.11" }, //<= Notice here single item in list with comma(,) separated
new List<string> { "2.11, 3.11, 4.11" },
new List<string> { "4.11, 5.11, 6.11" }
};
So you need to first split your string in inner list with comma(,
) to get each item as separate string,
var list = myList
.SelectMany(x => x
.Select(y => y.Split(',')
.Select(z => z.Trim())
.ToList()))
.ToList();
Then you can make .Zip
on all above 3 list by
var results = list[0]
.Zip(list[1], (a, b) => double.Parse(a) + double.Parse(b))
.Zip(list[2], (x, y) => (x + double.Parse(y)) / 3)
.ToList();
//------------------Print the result---------------
foreach (var item in results)
{
Console.WriteLine(Math.Round(item, 2));
}
How .Zip
works?
Lets take all columns value on index 0.
a = "1.11"
b = "2.11"
Then 1st Zip
result will be =>
double.Parse(a) + double.Parse(b)
= 1.11 + 2.11
= 3.22
So for 2nd .Zip
the x
now be the result of the above 1st .Zip
that means
x = 3.22
y = "4.11"
Then 2nd Zip
result will be =>
(x + double.Parse(y)) / 3
= (3.22 + 4.11) / 3
= 2.44
So for Average
of your values at column index 0 => 2.44
In above,
- list[0] : 1st list in your list of list of string.
- list[1] : 2nd list in your list of list of string.
- list[2] : 3rd list in your list of list of string.
Output: (From Console)
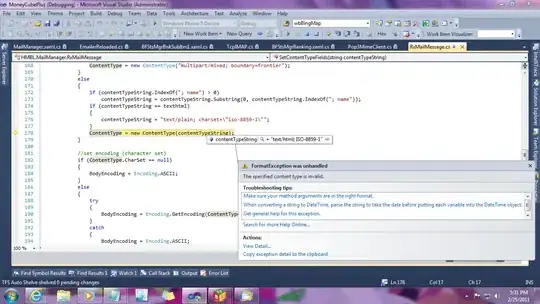