why we use WebDriver driver = new FirefoxDriver()
in script see below
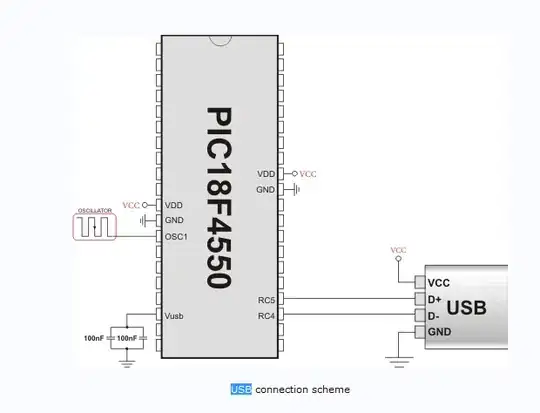
Following are the few points based on the above image.
-SearchContext is the super most interface in selenium, which is extended by another interface called WebDriver.
-All the abstract methods of SearchContext and WebDriver interfaces are implemented in RemoteWebDriver class.
-All the browser related classes such as FirefoxDriver, ChromeDriver etc., extends the RemoteWebdriver class.
What is WebDriver?
WebDriver is an interface provided by Selenium WebDriver. As we know that interfaces in Java are the collection of constants and abstract methods(methods without any implementation). The WebDriver interface serves as a contract that each browser specific implementation like ChromeDriver, FireFoxDriver must follow. The WebDriver interface declares methods like get(), navigate(), close(), sendKeys() etc. and the developers of the browser specific drivers implement these methods to get the stuff automated.
Take for example the ChromeDriver, it is developed by the guys from Chromium team, the developers of the Selenium project don't have to worry about the implementation details of these drivers.
WebDriver driver = new FirefoxDriver();
Having a reference variable of type WebDriver allows us to assign the driver object to different browser specific drivers. Thus allowing multi-browser testing by assigning the driver object to any of the desired browser.
For more information check following links:-
WebDriver driver = new FirefoxDriver() – Why we write in Selenium Scripts
How does it work?
In Selenium , WebDriver is a interface.
FirefoxDriver is a class. It implements all the methods of WebDriver interface.