Note that this is a workaround only (since there is no official way):
You can use Application.CommandBars.ExecuteMso
to run any buttons on the ribbon. Eg Application.CommandBars.ExecuteMso "EquationProfessional"
to convert the formula into professional style.
See Are the command codes for ExecuteMso documented? for how to get the identifiers.
Here are some ideas. Note that you need to create an equation shape object and call it Textfeld 1
before you run this codes. I didn't manage to create one via VBA but you can at least change the content of an existing equation.
TestWriteFormulaA
writes the formula
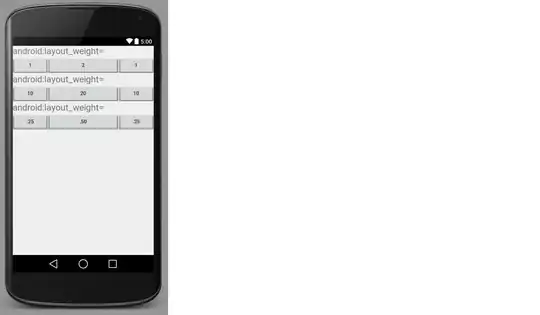
TestWriteFormulaB
writes the formula
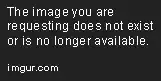
MakeEquationLinear
converts eg the FormulaA into the linear form
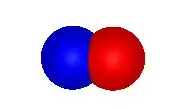
MakeEquationProfessional
converts it back to the professional form
GenerateAscWFromString
generates the ChrW
combination of a equation.
Note that the equation can only be changed in the linear form MyEquation.DrawingObject.Text =
. So make sure it is linear befor you generate the ChrW
combination.
Note that you can convert most signs into commands like \sum
for the sum sign ∑.
So instead of the Chrw
combination you can use eg
MyEquation.DrawingObject.Text = "(x+a)^n=\sum_(k=0)^n (n¦k)x^k a^(n-k)"
to write FormulaA.
Here you can find an inoffical documentation about the equation commands:
http://www.iun.edu/~mathiho/useful/Equation%20Editor%20Shortcut%20Commands.pdf
Option Explicit
' write a formula A
Public Sub TestWriteFormulaA()
Dim MyEquation As Shape
Set MyEquation = ThisWorkbook.Worksheets("Sheet1").Shapes("Textfeld 1")
MakeEquationLinear MyEquation
MyEquation.DrawingObject.Text = ChrW(40) & ChrW(-10187) & ChrW(-9115) & ChrW(43) & ChrW(-10187) & ChrW(-9138) & ChrW(41) & ChrW(94) & ChrW(-10187) & ChrW(-9125) & ChrW(61) & ChrW(8721) & ChrW(50) & ChrW(52) & ChrW(95) & ChrW(40) & ChrW(-10187) & ChrW(-9128) & ChrW(61) & ChrW(48) & ChrW(41) & ChrW(94) & ChrW(-10187) & ChrW(-9125) & ChrW(9618) & ChrW(12310) & ChrW(40) & ChrW(-10187) & ChrW(-9125) & ChrW(166) & ChrW(-10187) & ChrW(-9128) & ChrW(41) & ChrW(32) & ChrW(-10187) & ChrW(-9115) & ChrW(94) & ChrW(-10187) & ChrW(-9128) & ChrW(32) & ChrW(-10187) & ChrW(-9138) & ChrW(94) & ChrW(40) & ChrW(-10187) & ChrW(-9125) & ChrW(8722) & ChrW(-10187) & ChrW(-9128) & ChrW(41) & ChrW(32) & ChrW(12311)
'same as below which was converted manually to the command structure
'MyEquation.DrawingObject.Text = "(x+a)^n=\sum_(k=0)^n (n¦k)x^k a^(n-k)"
MakeEquationProfessional MyEquation
End Sub
' write another forumla B
Public Sub TestWriteFormulaB()
Dim MyEquation As Shape
Set MyEquation = ThisWorkbook.Worksheets("Sheet1").Shapes("Textfeld 1")
MakeEquationLinear MyEquation
MyEquation.DrawingObject.Text = ChrW(40) & ChrW(49) & ChrW(43) & ChrW(-10187) & ChrW(-9115) & ChrW(41) & ChrW(94) & ChrW(-10187) & ChrW(-9125) & ChrW(61) & ChrW(49) & ChrW(43) & ChrW(-10187) & ChrW(-9125) & ChrW(-10187) & ChrW(-9115) & ChrW(47) & ChrW(49) & ChrW(33) & ChrW(43) & ChrW(40) & ChrW(-10187) & ChrW(-9125) & ChrW(40) & ChrW(-10187) & ChrW(-9125) & ChrW(8722) & ChrW(49) & ChrW(41) & ChrW(32) & ChrW(-10187) & ChrW(-9115) & ChrW(94) & ChrW(50) & ChrW(41) & ChrW(47) & ChrW(50) & ChrW(33) & ChrW(43) & ChrW(8230)
MakeEquationProfessional MyEquation
End Sub
'get the ChrW combination of an equation in the immediate window
Public Sub TestGetChrWFromEquation()
Dim MyEquation As Shape
Set MyEquation = ThisWorkbook.Worksheets("Sheet1").Shapes("Textfeld 1")
MakeEquationLinear MyEquation
GenerateAscWFromString MyEquation.DrawingObject.Text
MakeEquationProfessional MyEquation
End Sub
Public Sub MakeEquationLinear(ByVal Equation As Shape)
Dim OriginalSheet As Object
If Equation.Parent.Name <> ActiveSheet.Name Then
Set OriginalSheet = ActiveSheet
Equation.Parent.Activate
End If
Equation.Select
Application.CommandBars.ExecuteMso "EquationLinearFormat"
If Not OriginalSheet Is Nothing Then OriginalSheet.Activate
End Sub
Public Sub MakeEquationProfessional(ByVal Equation As Shape)
Dim OriginalSheet As Object
If Equation.Parent.Name <> ActiveSheet.Name Then
Set OriginalSheet = ActiveSheet
Equation.Parent.Activate
End If
Equation.Select
Application.CommandBars.ExecuteMso "EquationProfessional"
If Not OriginalSheet Is Nothing Then OriginalSheet.Activate
End Sub
Public Sub GenerateAscWFromString(ByVal InputString As String)
Dim OutputString As String
Dim ChrIdx As Long
For ChrIdx = 1 To Len(InputString)
OutputString = OutputString & IIf(OutputString <> vbNullString, " & ", "") & "ChrW(" & AscW(Mid$(InputString, ChrIdx, 1)) & ")"
Next ChrIdx
Debug.Print OutputString
End Sub