Solution:
You can use filter
with a local Set
object. By comparing the stringified objects and the size
property before and after trying to add to the Set
, you can return a unique array by asking if the two numbers are not equal.
Code:
place_array.filter(v => b.size != b.add(JSON.stringify(v)).size, b = new Set());
Why use this code?
Unlike many of the other answers it relies on a singular pass through the array using the filter iterator. This means that it's more performant than multiple loops through an array. It also uses only localized variables, meaning that their is no global clutter and, since the filter
method is functional, absolutely no side effects.
Note: There is always a hit to performance when using a Set
Object in any situation. This comes down to Browser internals, however, when finding unique Objects/Arrays the stringify
into a Set
method is likely the best solution. Though with incredibly small data-sets you may find it practical to use an Array or build your own task-specific Object.
Working Example:
let place_array = [{
"places": "NEW YORK",
"locations": "USA"
},
{
"places": "MONTREAL",
"locations": "QUEBEC",
"country": "Canada"
},
{
"places": "MONTREAL",
"locations": "QUEBEC",
"country": "Canada"
},
];
let result = place_array.filter(v => b.size != b.add(JSON.stringify(v)).size, b = new Set());
console.log(result);
Alternative Solution:
You can use filter
with a local Array
object. By determining whether or not the stringified objects are in the Array already and pushing if they're not, you can return a unique array by asking if the stringified object is not included in the array.
Code:
place_array.filter(v => !b.includes(s(v)) && !!b.push(s(v)), s = JSON.stringify, b = []);
Why use this code?
Effective though the Set
object may be, it is ultimately slower due to backend processes than utilizing an Array
object. You can prove this quite easily by running a test like a jsperf: https://jsperf.com/set-vs-ray-includes-battle/1
The Results of a Test:
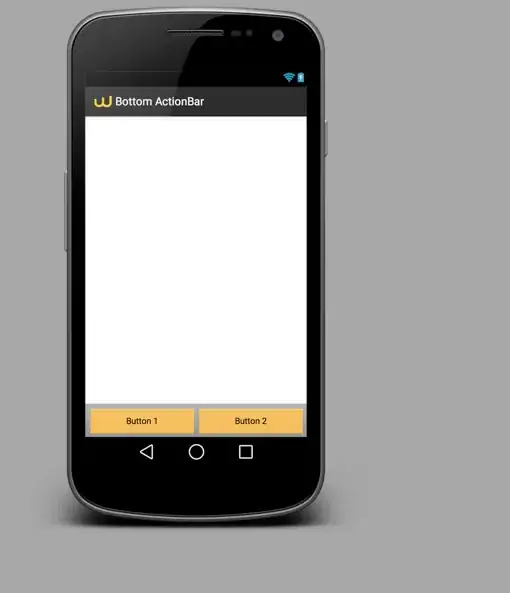
Working Example:
let place_array = [{
"places": "NEW YORK",
"locations": "USA"
},
{
"places": "MONTREAL",
"locations": "QUEBEC",
"country": "Canada"
},
{
"places": "MONTREAL",
"locations": "QUEBEC",
"country": "Canada"
},
];
let result = place_array.filter(v => !b.includes(s(v)) && !!b.push(s(v)), s = JSON.stringify, b = []);
console.log(result);