For this you need to use an emission
instance property that defines the color emitted by each point on a surface.
You can use an emissive map texture to simulate parts of a surface that glow with their own light. SceneKit does not treat the material as a light source—rather, the emission property determines colors for a material independent of lighting. (To create an object that appears to glow, you may wish to combine a geometry with an emissive map and additional SCNLight objects added to the scene.)
var emission: SCNMaterialProperty { get }
You can find emission
property in any available SceneKit's shader Constant
, Lambert
, Blinn
, Phong
or Physically Based
.
let earth = SCNNode(geometry: SCNSphere(radius: 5))
earth.geometry?.firstMaterial?.emission.contents = UIImage(named: "emissionMap.png")
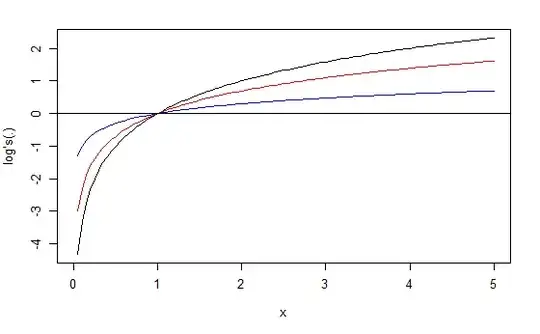
And, of course, if you need to create a square emissionMap.png
texture (a.k.a. UV Map) you should make it in Autodesk Maya or in Autodesk 3dsMax or in Maxon Cinema4D, etc. Such maps could be 512x512, 1024x1024, 2048x2048, etc.
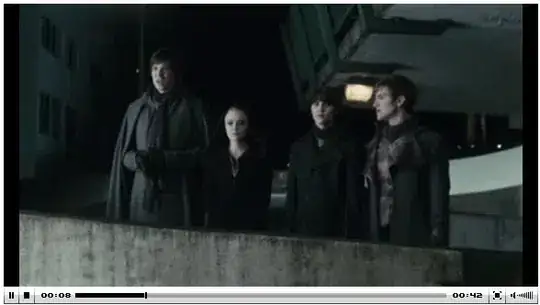