Kony supports Preprocessor Directives such as #ifdef
much like the C compiler's preprocessors. Since Kony projects are written in Javascript, these statements must be added in the form of special comments in order not to break the Javascript syntax. So for example #ifdef
becomes //#ifdef
.
These directives can be used to write code which gets built into the application or not depending on the host OS. So I've solved this by writing this:
var channel;
//#ifdef PLATFORM_NATIVE_IOS
channel = "ios"
//#endif
//#ifdef PLATFORM_NATIVE_ANDROID
channel = "android"
//#endif
And then writing the rest of my logic based on the value of my channel
variable.
For a full list of the macros defined which you can use in these //#ifdef
statements you can look at the first few lines in the kony_sdk.js
module created by default in every Kony Visualizer project.
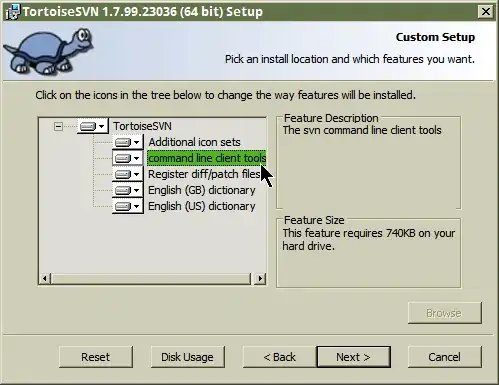