You can create programmatically by using below code:
CGRect someRect = CGRectMake(0.0, 0.0, 100.0, 30.0);
DKTextField* textField = [[DKTextField alloc] initWithFrame:someRect];
textField.padding = 1.0
[self.view addSubview:textField];
Use Below Classes for it
DKTextField.h
#import <UIKit/UIKit.h>
@interface DKTextField : UITextField
@property (nonatomic) IBInspectable CGFloat padding;
@end
DKTextField.m
#import "DKTextField.h"
IB_DESIGNABLE
@implementation DKTextField
@synthesize padding;
-(CGRect)textRectForBounds:(CGRect)bounds{
return CGRectInset(bounds, padding, padding);
}
-(CGRect)editingRectForBounds:(CGRect)bounds{
return [self textRectForBounds:bounds];
}
@end
You just have to give your selected UITextField
to DKTextField
Class
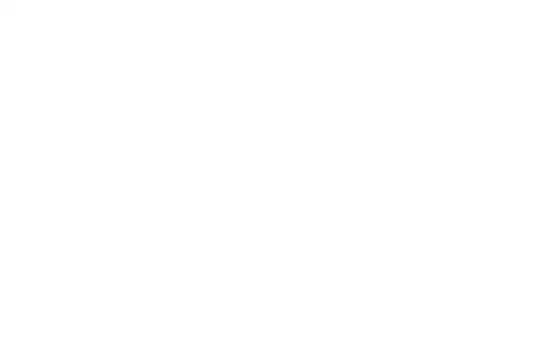
Whatever the padding you want just add it like below image.
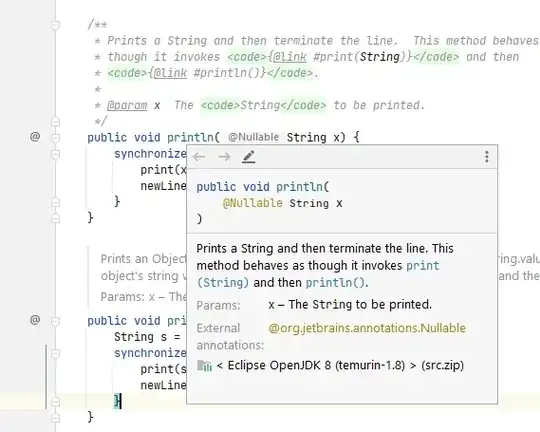