This is a small sample program to write an 8-bit graylevel image into a Windows BMP file:
#include <stdio.h>
typedef unsigned char Byte;
int writeBMPGray8(FILE *f, int w, int h, const Byte *data)
{
unsigned bytesPerRow = (w + 3) & ~3; // align to 4 bytes (requirement)
unsigned size
= 14 // Bitmap file header size
+ 12 // DIB header size
+ 256 * 3; // palette size
unsigned gap = size;
size = (size + 3) & ~3; // align to 4 bytes (requirement)
gap = size - gap; // get size of gap between end of headers and raw data
unsigned offs = size; // store offset of raw data
size += h * bytesPerRow; // bitmap data size in file
/* write Bitmap file header (14 bytes) */
{ const Byte buffer[14] = {
'B', 'M', // magic code
size & 0xff, size >> 8 & 0xff, size >> 16 & 0xff, size >> 24 & 0xff, // size of BMP file in bytes
0, 0, // reserved
0, 0, // reserved
offs & 0xff, offs >> 8 & 0xff, offs >> 16 & 0xff, offs >> 24 & 0xff // starting offset of pixel data
};
if (fwrite(buffer, sizeof buffer, 1, f) != 1) return -1; // ERROR!
}
/* write DIB header (12 bytes) */
{ const Byte buffer[12] = {
12, 0, 0, 0, // size of this header
w & 0xff, w >> 8 & 0xff, // bitmap width in pixels
h & 0xff, h >> 8 & 0xff, // bitmap height in pixels
1, 0, // number of color planes, must be 1
8, 0 // number of bits per pixel
};
if (fwrite(buffer, sizeof buffer, 1, f) != 1) return -1; // ERROR!
}
/* write color palette (3 * 256 bytes) */
for (int i = 0; i < 256; ++i) { // make a gray level palette
Byte buffer[3] = { i, i, i };
if (fwrite(buffer, sizeof buffer, 1, f) != 1) return -1; // ERROR!
}
/* write gap (to align start address of raw data with 4 */
for (int i = 0; i < gap; ++i) {
if (fputc(0, f) < 0) return -1; // ERROR!
}
/* write raw data */
for (int y = 0; y < h; ++y) { // for all rows
int x = 0;
for (; x < w; ++x) { // for all columns
if (fputc(*data++, f) < 0) return -1; // ERROR!
}
// write row padding
for (; x < bytesPerRow; ++x) {
if (fputc(0, f) < 0) return -1; // ERROR!
}
}
/* done */
return 0;
}
int main()
{
/* a sample image 6 x 8, gray level */
enum { w = 6, h = 8 };
const Byte imgRaw[w * h] = {
0x00, 0x30, 0x60, 0x90, 0xc0, 0xf0,
0x02, 0x32, 0x62, 0x92, 0xc2, 0xf2,
0x04, 0x34, 0x64, 0x94, 0xc4, 0xf4,
0x06, 0x36, 0x66, 0x96, 0xc6, 0xf6,
0x08, 0x38, 0x68, 0x98, 0xc8, 0xf8,
0x0a, 0x3a, 0x6a, 0x9a, 0xca, 0xfa,
0x0c, 0x3c, 0x6c, 0x9c, 0xcc, 0xfc,
0x0e, 0x3e, 0x6e, 0x9e, 0xce, 0xfe
};
FILE *f = fopen("test.bmp", "wb");
if (!f) return 1; // ERROR!
if (writeBMPGray8(f, w, h, imgRaw)) return 1; // ERROR!
if (fclose(f)) return 1; // ERROR!
return 0; // success
}
The sample image provides some kind of gradients horizontally and vertically. I've chosen a width of 6 intentionally to check/show that row alignment is done properly.
The implementation is based on the description in Wikipedia BMP file format.
To keep it short, I encoded the simplest format – the ancient BITMAPCOREHEADER
of Windows 2.0 and OS/2 1.x. (MS Paint can load this as well as the Windows 10 preview. I tested with GIMP which loaded as well without any complaints.)
This is how it looks in GIMP:
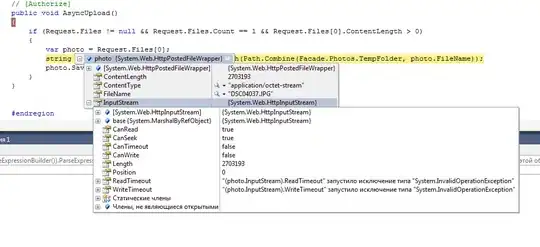